Java如何将excel中的某一列内容按照逗号分隔分成三列
时间: 2024-10-09 12:08:06 浏览: 37
在Java中,我们可以使用Apache POI库来处理Excel文件,特别是HSSFWorkbook或XSSFWorkbook类。对于将Excel的一列内容按照逗号分隔成三列,你可以按照以下步骤操作:
1. 导入所需库:
```java
import org.apache.poi.ss.usermodel.*;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
```
2. 读取Excel文件并获取需要的行数据:
```java
FileInputStream file = new FileInputStream("your_file_path.xlsx");
Workbook workbook = new XSSFWorkbook(file);
Sheet sheet = workbook.getSheetAt(0); // 假设第一张工作表
Iterator<Row> rowIterator = sheet.iterator();
```
3. 遍历每一行,对指定列的内容进行分割:
```java
while (rowIterator.hasNext()) {
Row row = rowIterator.next();
Cell cell = row.getCell(columnIndex); // 指定目标列的索引,从0开始计数
String content = cell.getStringCellValue();
if (content != null) {
String[] parts = content.split(",");
if (parts.length >= 3) {
// 将三个部分分别放入新的cell或者新的一行,这里仅示例赋值
Cell cell1 = row.createCell(columnIndex + 1);
cell1.setCellValue(parts[0]);
Cell cell2 = row.createCell(columnIndex + 2);
cell2.setCellValue(parts[1]);
Cell cell3 = row.createCell(columnIndex + 3);
cell3.setCellValue(parts[2]);
}
}
}
```
4. 保存修改后的文件:
```java
try {
FileOutputStream outputStream = new FileOutputStream("output_file.xlsx");
workbook.write(outputStream);
workbook.close();
outputStream.close();
} catch (Exception e) {
e.printStackTrace();
}
```
阅读全文
相关推荐

















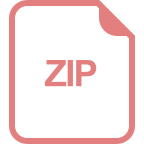
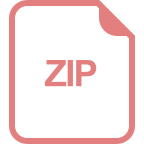