使用C++实现布丰投针法
时间: 2024-12-23 08:16:48 浏览: 9
在C++中实现布丰投针法(Buffon's Needle Problem)可以按照以下步骤进行:
1. 定义常量:设置针的长度`double needle_length`,以及平行线之间的间距`double gap`。针长一般选择为`needle_length = 1.0`,为了简单起见。
```cpp
const double needle_length = 1.0;
const double gap = 2.0; // 根据题目描述,针长是平行线间距的一半,这里假设两倍针长作为间距
```
2. 创建随机投掷函数:生成一个随机的针投掷方向(通常通过`<random>`库),并计算针中心点到最近平行线的距离。
```cpp
#include <random>
double random_direction() {
static std::default_random_engine generator(std::chrono::system_clock::now().time_since_epoch().count());
static std::uniform_real_distribution<double> distribution(0., 1.);
return distribution(generator);
}
double distance_to_nearest_parallel(double x) {
return needle_length * std::sin(std::acos(x)); // 根据题意,如果x在[0,1]范围内,则表示角度,sin(acos(x))即为正弦乘以夹角
}
```
3. 投掷循环:重复投掷针并统计相交次数,然后计算概率。
```cpp
int num_intersections = 0;
for (int i = 0; i < total_trials; ++i) {
double angle = random_direction();
double intersection = distance_to_nearest_parallel(angle);
if (intersection < gap / 2.0) { // 判断是否相交
num_intersections++;
}
}
// 计算圆周率
double pi_approximation = (2.0 * needle_length) / (gap * static_cast<double>(num_intersections) / total_trials);
```
4. 输出结果:打印计算出的π近似值。
```cpp
std::cout << "Estimated value of Pi: " << pi_approximation << std::endl;
```
请注意,由于这是一个模拟实验,实际运行时`total_trials`应足够大,以便得到更准确的结果。这个算法并不能保证每次都能得到精确的π值,但随着试验次数增加,平均值会趋向于π。
阅读全文
相关推荐
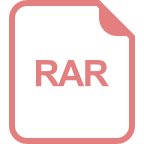
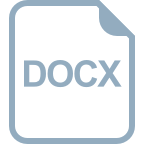

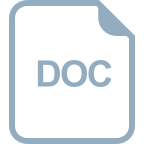
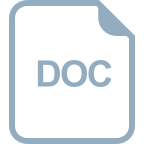
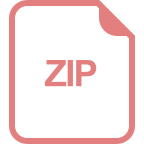
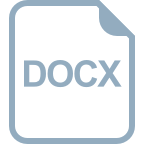
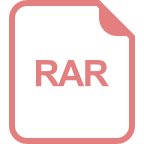
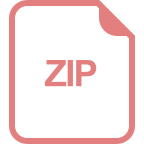
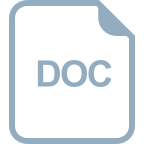
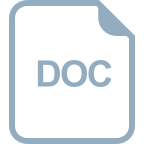


