用java和MySQL做一个图书管理系统
时间: 2023-10-29 07:05:18 浏览: 84
好的,下面是一个简单的图书管理系统的Java代码,使用MySQL作为数据库:
首先,需要创建一个名为“library”的MySQL数据库,其中包含一个名为“books”的表,表中包含以下字段:id(自增长的图书ID)、title(图书名)、author(作者)、publisher(出版社)、year(出版年份)、isbn(ISBN号码)、quantity(库存数量)。
接下来是Java代码:
```
import java.sql.*;
public class LibrarySystem {
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/library";
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
Statement stmt = null;
try {
Class.forName(JDBC_DRIVER);
conn = DriverManager.getConnection(DB_URL, USER, PASS);
stmt = conn.createStatement();
String sql;
// 创建books表
sql = "CREATE TABLE IF NOT EXISTS books " +
"(id INTEGER not NULL AUTO_INCREMENT, " +
" title VARCHAR(255), " +
" author VARCHAR(255), " +
" publisher VARCHAR(255), " +
" year INTEGER, " +
" isbn VARCHAR(13), " +
" quantity INTEGER, " +
" PRIMARY KEY ( id ))";
stmt.executeUpdate(sql);
// 添加书籍
sql = "INSERT INTO books (title, author, publisher, year, isbn, quantity) " +
"VALUES ('Java Programming', 'John Smith', 'ABC Press', 2020, '1234567890123', 10)";
stmt.executeUpdate(sql);
// 更新库存数量
sql = "UPDATE books SET quantity = 5 WHERE title = 'Java Programming'";
stmt.executeUpdate(sql);
// 查询书籍
sql = "SELECT * FROM books WHERE author = 'John Smith'";
ResultSet rs = stmt.executeQuery(sql);
while (rs.next()) {
int id = rs.getInt("id");
String title = rs.getString("title");
String author = rs.getString("author");
String publisher = rs.getString("publisher");
int year = rs.getInt("year");
String isbn = rs.getString("isbn");
int quantity = rs.getInt("quantity");
System.out.println("ID: " + id);
System.out.println("Title: " + title);
System.out.println("Author: " + author);
System.out.println("Publisher: " + publisher);
System.out.println("Year: " + year);
System.out.println("ISBN: " + isbn);
System.out.println("Quantity: " + quantity);
}
rs.close();
stmt.close();
conn.close();
} catch (SQLException se) {
se.printStackTrace();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
}
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
}
}
}
```
这个程序可以执行以下操作:
1. 创建名为“library”的MySQL数据库和“books”表
2. 向表中添加一本书
3. 更新书的库存数量
4. 查询一本书
你可以根据自己的需求进行修改和扩展。
相关推荐
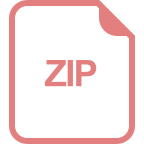
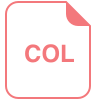
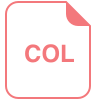
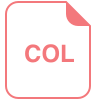
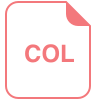
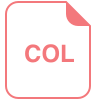









