帮我找一些有关python内置对象,运算符,表达式,关键字的基础练习题
时间: 2023-05-23 11:01:18 浏览: 61
当然可以,以下是一些Python基础练习题与答案:
1. 写一个程序,实现两个整数相加,要求输入两个整数并打印结果。
答案:
```python
num1 = int(input("Enter the first number: "))
num2 = int(input("Enter the second number: "))
result = num1 + num2
print(f"The sum of {num1} and {num2} is {result}")
```
2. 写一个程序,输入半径并计算圆的面积和周长。
答案:
```python
radius = float(input("Enter the radius: "))
area = 3.14 * radius ** 2
circumference = 2 * 3.14 * radius
print(f"The area of the circle is {area} square units")
print(f"The circumference of the circle is {circumference} units")
```
3. 写一个程序,输入一个浮点数并将其转换为整数输出。
答案:
```python
num = float(input("Enter a float number: "))
integer = int(num)
print(f"The integer value of {num} is {integer}")
```
4. 写一个程序,输入两个字符串并将它们连接起来输出。
答案:
```python
str1 = input("Enter the first string: ")
str2 = input("Enter the second string: ")
result = str1 + " " + str2
print(f"The concatenated string is '{result}'")
```
5. 写一个程序,输入一个数字并判断它是否为正数、负数或零。
答案:
```python
num = float(input("Enter a number: "))
if num > 0:
print("The number is positive")
elif num < 0:
print("The number is negative")
else:
print("The number is zero")
```
希望这些练习题对你有所帮助!
相关推荐
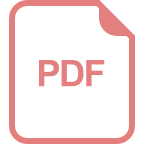
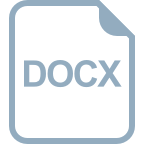
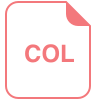
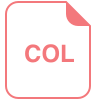
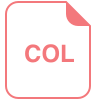
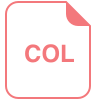
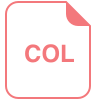









