wgs84转cgcs2000 java_CGCS2000坐标系与WGS84的相互投影转换
时间: 2023-07-29 09:07:03 浏览: 255
要将坐标从WGS84投影到CGCS2000或从CGCS2000投影到WGS84,需要使用适当的转换参数。以下是Java代码示例,演示如何将WGS84坐标系转换为CGCS2000坐标系:
```java
import org.geotools.referencing.CRS;
import org.geotools.referencing.crs.DefaultGeographicCRS;
import org.geotools.referencing.operation.DefaultCoordinateOperationFactory;
import org.geotools.referencing.operation.MathTransform;
public class WGS84ToCGCS2000 {
public static void main(String[] args) throws Exception {
// Define WGS84 CRS
String wgs84 = "EPSG:4326";
// Define CGCS2000 CRS
String cgcs2000 = "EPSG:4490";
// Define WGS84 coordinates
double lat = 39.9;
double lon = 116.3;
// Create coordinate operation factory
DefaultCoordinateOperationFactory factory = new DefaultCoordinateOperationFactory();
// Create math transform from WGS84 to CGCS2000
MathTransform transform = factory.createOperation(
CRS.decode(wgs84),
CRS.decode(cgcs2000),
null).getMathTransform();
// Transform WGS84 coordinates to CGCS2000
double[] source = new double[]{lon, lat};
double[] target = new double[2];
transform.transform(source, 0, target, 0, 1);
// Print CGCS2000 coordinates
System.out.println("CGCS2000 coordinates: " + target[0] + ", " + target[1]);
}
}
```
同样,如果要将坐标从CGCS2000投影到WGS84,可以使用以下代码示例:
```java
import org.geotools.referencing.CRS;
import org.geotools.referencing.crs.DefaultGeographicCRS;
import org.geotools.referencing.operation.DefaultCoordinateOperationFactory;
import org.geotools.referencing.operation.MathTransform;
public class CGCS2000ToWGS84 {
public static void main(String[] args) throws Exception {
// Define WGS84 CRS
String wgs84 = "EPSG:4326";
// Define CGCS2000 CRS
String cgcs2000 = "EPSG:4490";
// Define CGCS2000 coordinates
double x = 433067.03;
double y = 4371345.63;
// Create coordinate operation factory
DefaultCoordinateOperationFactory factory = new DefaultCoordinateOperationFactory();
// Create math transform from CGCS2000 to WGS84
MathTransform transform = factory.createOperation(
CRS.decode(cgcs2000),
CRS.decode(wgs84),
null).getMathTransform();
// Transform CGCS2000 coordinates to WGS84
double[] source = new double[]{x, y};
double[] target = new double[2];
transform.transform(source, 0, target, 0, 1);
// Print WGS84 coordinates
System.out.println("WGS84 coordinates: " + target[1] + ", " + target[0]);
}
}
```
请注意,这些示例代码需要使用GeoTools库。您可以在https://docs.geotools.org/latest/userguide/library/referencing/install.html网站上找到有关如何安装GeoTools的更多信息。
阅读全文
相关推荐
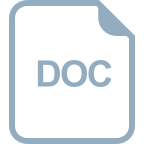
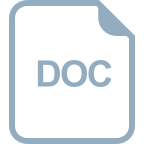
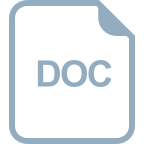
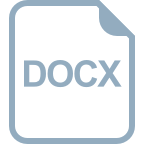
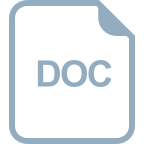











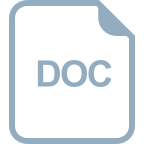