2、创建jdbc项目,实现数据库连接,并完成如下功能: 1)输入一个部门]的信息,完成部门的添加; 2)根据部门编号针对部门门名称进行修改的功能:输入部门编号和修改 名称的修改! 3)输入一个员工的信息,完成员工的添加。 4)完成如下查询: 4.1)查询所有员工信息; 4.2)输入姓名,查询员工信息; 3、创建jdbc项目,使用逻辑三层结构,把第2题的功能重新实现一遍;
时间: 2023-12-03 21:42:07 浏览: 31
对于第一部分,需要先创建一个数据库,在其中创建两个表,分别为dept和employee。dept表包含dept_id和dept_name两个字段,employee表包含emp_id,emp_name,dept_id三个字段。然后在Java中使用JDBC连接数据库,实现对部门和员工的添加、修改和查询操作。
以下是一个简单的示例代码,仅供参考:
```java
import java.sql.*;
public class JdbcDemo {
private static final String URL = "jdbc:mysql://localhost:3306/db_name";
private static final String USERNAME = "username";
private static final String PASSWORD = "password";
public static void main(String[] args) {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
// 添加部门
String sql = "insert into dept(dept_id, dept_name) values (?, ?)";
ps = conn.prepareStatement(sql);
ps.setInt(1, 1);
ps.setString(2, "Sales");
ps.executeUpdate();
// 修改部门名称
sql = "update dept set dept_name = ? where dept_id = ?";
ps = conn.prepareStatement(sql);
ps.setString(1, "Marketing");
ps.setInt(2, 1);
ps.executeUpdate();
// 添加员工
sql = "insert into employee(emp_id, emp_name, dept_id) values (?, ?, ?)";
ps = conn.prepareStatement(sql);
ps.setInt(1, 1);
ps.setString(2, "John");
ps.setInt(3, 1);
ps.executeUpdate();
// 查询所有员工信息
sql = "select emp_id, emp_name, dept_name from employee inner join dept on employee.dept_id = dept.dept_id";
ps = conn.prepareStatement(sql);
rs = ps.executeQuery();
while (rs.next()) {
System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
}
// 根据姓名查询员工信息
sql = "select emp_id, emp_name, dept_name from employee inner join dept on employee.dept_id = dept.dept_id where emp_name = ?";
ps = conn.prepareStatement(sql);
ps.setString(1, "John");
rs = ps.executeQuery();
while (rs.next()) {
System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
}
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (rs != null) rs.close();
if (ps != null) ps.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
}
```
对于第二部分,需要在第一部分的基础上进行重构,实现逻辑三层结构。这里简单介绍一下逻辑三层结构的组成:
- 数据访问层(DAO):负责数据库的读写操作,将数据保存到数据库或从数据库中读取数据并返回给上层。
- 业务逻辑层(Service):负责对业务逻辑的处理,将数据传递给DAO层进行保存或读取,同时对数据进行加工和处理。
- 表现层(Presentation):负责展示数据,将数据展示给用户或接收用户的输入,并调用Service层对数据进行处理。
以下是一个简单的示例代码,仅供参考:
1. 数据访问层(DAO)
```java
import java.sql.*;
public class DeptDAO {
private static final String URL = "jdbc:mysql://localhost:3306/db_name";
private static final String USERNAME = "username";
private static final String PASSWORD = "password";
public void addDept(int deptId, String deptName) {
Connection conn = null;
PreparedStatement ps = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
String sql = "insert into dept(dept_id, dept_name) values (?, ?)";
ps = conn.prepareStatement(sql);
ps.setInt(1, deptId);
ps.setString(2, deptName);
ps.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (ps != null) ps.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public void updateDept(int deptId, String deptName) {
Connection conn = null;
PreparedStatement ps = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
String sql = "update dept set dept_name = ? where dept_id = ?";
ps = conn.prepareStatement(sql);
ps.setString(1, deptName);
ps.setInt(2, deptId);
ps.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (ps != null) ps.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public void addEmployee(int empId, String empName, int deptId) {
Connection conn = null;
PreparedStatement ps = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
String sql = "insert into employee(emp_id, emp_name, dept_id) values (?, ?, ?)";
ps = conn.prepareStatement(sql);
ps.setInt(1, empId);
ps.setString(2, empName);
ps.setInt(3, deptId);
ps.executeUpdate();
} catch (Exception e) {
e.printStackTrace();
} finally {
try {
if (ps != null) ps.close();
if (conn != null) conn.close();
} catch (SQLException e) {
e.printStackTrace();
}
}
}
public ResultSet getAllEmployees() {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
String sql = "select emp_id, emp_name, dept_name from employee inner join dept on employee.dept_id = dept.dept_id";
ps = conn.prepareStatement(sql);
rs = ps.executeQuery();
} catch (Exception e) {
e.printStackTrace();
}
return rs;
}
public ResultSet getEmployeeByName(String empName) {
Connection conn = null;
PreparedStatement ps = null;
ResultSet rs = null;
try {
Class.forName("com.mysql.jdbc.Driver");
conn = DriverManager.getConnection(URL, USERNAME, PASSWORD);
String sql = "select emp_id, emp_name, dept_name from employee inner join dept on employee.dept_id = dept.dept_id where emp_name = ?";
ps = conn.prepareStatement(sql);
ps.setString(1, empName);
rs = ps.executeQuery();
} catch (Exception e) {
e.printStackTrace();
}
return rs;
}
}
```
2. 业务逻辑层(Service)
```java
import java.sql.ResultSet;
import java.sql.SQLException;
public class DeptService {
private DeptDAO deptDAO;
public DeptService() {
deptDAO = new DeptDAO();
}
public void addDept(int deptId, String deptName) {
deptDAO.addDept(deptId, deptName);
}
public void updateDept(int deptId, String deptName) {
deptDAO.updateDept(deptId, deptName);
}
public void addEmployee(int empId, String empName, int deptId) {
deptDAO.addEmployee(empId, empName, deptId);
}
public void printAllEmployees() {
ResultSet rs = deptDAO.getAllEmployees();
try {
while (rs.next()) {
System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
public void printEmployeeByName(String empName) {
ResultSet rs = deptDAO.getEmployeeByName(empName);
try {
while (rs.next()) {
System.out.println(rs.getInt(1) + " " + rs.getString(2) + " " + rs.getString(3));
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
3. 表现层(Presentation)
```java
import java.util.Scanner;
public class Main {
private static DeptService deptService;
public static void main(String[] args) {
deptService = new DeptService();
Scanner scanner = new Scanner(System.in);
while (true) {
System.out.println("1. 添加部门");
System.out.println("2. 修改部门名称");
System.out.println("3. 添加员工");
System.out.println("4. 查询所有员工信息");
System.out.println("5. 根据姓名查询员工信息");
System.out.println("6. 退出");
int choice = scanner.nextInt();
if (choice == 1) {
System.out.println("请输入部门编号和名称,以空格分隔:");
int deptId = scanner.nextInt();
String deptName = scanner.next();
deptService.addDept(deptId, deptName);
} else if (choice == 2) {
System.out.println("请输入部门编号和新名称,以空格分隔:");
int deptId = scanner.nextInt();
String deptName = scanner.next();
deptService.updateDept(deptId, deptName);
} else if (choice == 3) {
System.out.println("请输入员工编号、姓名和所在部门编号,以空格分隔:");
int empId = scanner.nextInt();
String empName = scanner.next();
int deptId = scanner.nextInt();
deptService.addEmployee(empId, empName, deptId);
} else if (choice == 4) {
deptService.printAllEmployees();
} else if (choice == 5) {
System.out.println("请输入员工姓名:");
String empName = scanner.next();
deptService.printEmployeeByName(empName);
} else if (choice == 6) {
break;
} else {
System.out.println("输入无效,请重新输入");
}
}
}
}
```
相关推荐
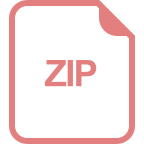
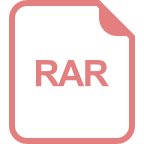
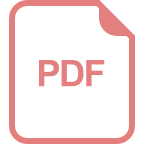














