在vue中使用echarts实现两条折线图
时间: 2023-11-06 10:04:03 浏览: 147
好的,以下是使用 Vue 和 Echarts 实现两条折线图的基本步骤:
1. 安装 Echarts
在 Vue 项目中使用 Echarts 需要先安装 Echarts,可以使用 npm 或 yarn 进行安装:
```bash
npm install echarts
```
或
```bash
yarn add echarts
```
2. 引入 Echarts
在需要使用 Echarts 的组件中引入 Echarts:
```javascript
import echarts from 'echarts'
```
3. 创建 Echarts 实例
在组件的 `mounted` 钩子函数中创建 Echarts 实例,并绑定到 Vue 实例的 `chart` 属性上:
```javascript
mounted() {
// 基于准备好的dom,初始化echarts实例
const chartDom = this.$refs.chart
const myChart = echarts.init(chartDom)
// 将echarts实例绑定到Vue实例的chart属性上
this.chart = myChart
}
```
4. 渲染折线图
定义数据和配置项,然后在组件的 `mounted` 钩子函数中使用 Echarts 的 `setOption` 方法来渲染折线图:
```javascript
mounted() {
const chartDom = this.$refs.chart
const myChart = echarts.init(chartDom)
this.chart = myChart
// 定义数据和配置项
const data = {
xData: ['周一', '周二', '周三', '周四', '周五', '周六', '周日'],
seriesData1: [120, 200, 150, 80, 70, 110, 130],
seriesData2: [80, 120, 110, 90, 60, 100, 80]
}
const option = {
tooltip: {
trigger: 'axis'
},
legend: {
data: ['数据1', '数据2']
},
xAxis: {
type: 'category',
boundaryGap: false,
data: data.xData
},
yAxis: {
type: 'value'
},
series: [{
name: '数据1',
type: 'line',
data: data.seriesData1
}, {
name: '数据2',
type: 'line',
data: data.seriesData2
}]
}
// 使用刚指定的配置项和数据显示图表。
myChart.setOption(option)
}
```
5. 在模板中渲染 Echarts 实例
最后,在模板中使用 `ref` 属性来引用 Echarts 容器,然后将容器渲染到页面中:
```html
<template>
<div>
<div ref="chart" style="width: 100%; height: 300px"></div>
</div>
</template>
```
这样就可以实现在 Vue 中使用 Echarts 渲染两条折线图了。
阅读全文
相关推荐
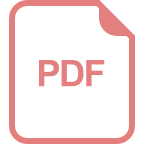
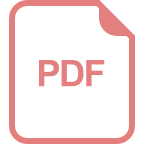
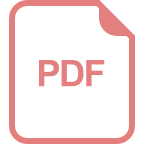
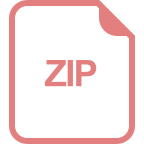
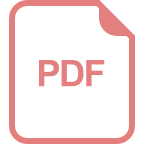
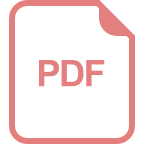
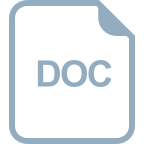
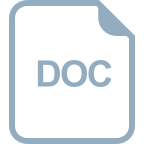




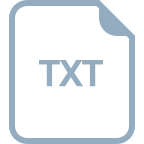
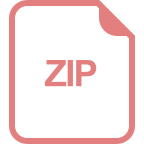
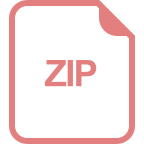
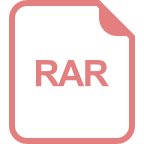
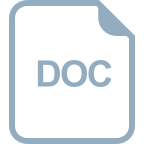

