springboot aop接口限流
时间: 2024-07-13 09:01:07 浏览: 58
Spring Boot 中的 AOP (Aspect Oriented Programming) 可以用来实现接口级别的限流功能,通常我们会结合第三方库如 Spring Cloud Gateway、Webrx 或者自定义拦截器来完成这个任务。以下是一个简单的概述:
1. 引入依赖:首先,在Spring Boot项目中添加限流库的依赖,比如 Spring Cloud Gateway 提供了 WebFlux 基于令牌桶(Token Bucket)的限流支持。
```xml
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-gateway</artifactId>
</dependency>
```
2. 定义限流规则:在配置类中设置限流策略,例如限制某个接口每秒的请求次数。Spring Cloud Gateway 使用 `RateLimiter` 来控制。
```java
@Bean
public RateLimiter myLimit(RateLimiterConfig config) {
// 设置限流参数,如每秒50次请求
return RateLimiter.of("my-limit", config.limitForPeriod(1, TimeUnit.SECONDS), false);
}
```
3. AOP 配置:创建一个切面(Aspect),利用 `@Around` 注解和 `RateLimiter` 对目标方法进行拦截,并在调用之前检查是否达到限流阈值。
```java
@Aspect
@Component
public class ApiRateLimitingAspect {
@Autowired
private RateLimiter myLimit;
@Around("@annotation(api)")
public Object limitApi(ProceedingJoinPoint joinPoint, Api api) throws Throwable {
if (!myLimit.tryAcquire()) {
throw new RateLimiterRejectedException("Exceeded rate limit");
}
// 执行原方法
return joinPoint.proceed();
}
// 如果你需要为每个接口定义不同的限流规则,可以使用注解来标记
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface Api {
String value() default "";
}
}
```
在这个例子中,我们假设有一个 `Api` 注解用于标记接口,然后在 `limitApi` 方法中对被该注解修饰的方法进行限流。
相关推荐
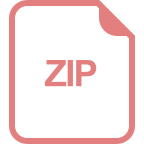
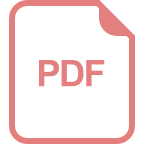
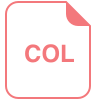
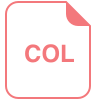
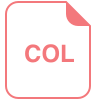
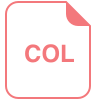
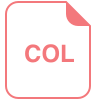
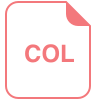
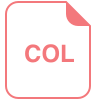
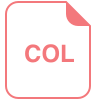
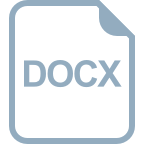