使用vue写一个轮播图
时间: 2024-06-09 13:12:20 浏览: 108
好的,以下是一个简单的使用 Vue 实现轮播图的例子:
```html
<template>
<div class="slider">
<ul class="slider-list" :style="{transform: 'translateX(' + translateX + 'px)'}">
<li class="slider-item" v-for="item in items" :key="item.id">
<img :src="item.imgUrl" alt="">
</li>
</ul>
<ul class="slider-dots">
<li class="slider-dot" v-for="(item, index) in items" :key="item.id" :class="{active: currentIndex === index}" @click="goTo(index)"></li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{id: 1, imgUrl: 'https://picsum.photos/id/1/600/400'},
{id: 2, imgUrl: 'https://picsum.photos/id/2/600/400'},
{id: 3, imgUrl: 'https://picsum.photos/id/3/600/400'},
],
currentIndex: 0,
translateX: 0,
timer: null,
interval: 2000,
duration: 500,
}
},
mounted() {
this.start()
},
methods: {
start() {
this.timer = setInterval(() => {
this.next()
}, this.interval)
},
next() {
this.goTo(this.currentIndex + 1)
},
goTo(index) {
if (index >= this.items.length) {
index = 0
} else if (index < 0) {
index = this.items.length - 1
}
this.currentIndex = index
this.translateX = -index * this.$refs.list.offsetWidth
clearInterval(this.timer)
setTimeout(() => {
this.start()
}, this.duration)
},
},
}
</script>
<style>
.slider {
position: relative;
width: 600px;
height: 400px;
overflow: hidden;
margin: 0 auto;
}
.slider-list {
display: flex;
align-items: center;
height: 100%;
transition: transform 0.5s ease-in-out;
}
.slider-item {
flex: 0 0 600px;
height: 400px;
}
.slider-dots {
position: absolute;
bottom: 20px;
left: 50%;
transform: translateX(-50%);
display: flex;
}
.slider-dot {
width: 10px;
height: 10px;
border-radius: 50%;
margin: 0 5px;
background-color: #ccc;
cursor: pointer;
}
.slider-dot.active {
background-color: #333;
}
</style>
```
在这个例子中,我们使用 `translateX` 属性来实现轮播图的滑动效果,使用定时器来自动播放,同时也支持手动切换。其中 `interval` 和 `duration` 分别表示自动播放的时间间隔和切换的动画时长,可以根据需要进行调整。
阅读全文
相关推荐
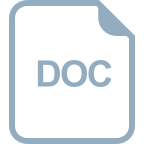
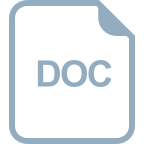
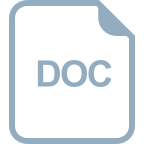


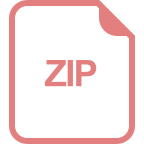
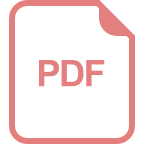











