用c语言实现共享栈和其基本操作
时间: 2023-10-11 12:09:54 浏览: 50
共享栈是两个栈公用一个数组的结构,可以在栈顶相遇后继续向下扩展。
以下是共享栈的基本操作的实现:
1. 初始化共享栈
```c
#define MAXSIZE 100 // 共享栈的最大长度
typedef struct {
int data[MAXSIZE];
int top1; // 栈1的栈顶指针
int top2; // 栈2的栈顶指针
} SharedStack;
// 初始化共享栈
void initSharedStack(SharedStack *s) {
s->top1 = -1;
s->top2 = MAXSIZE;
}
```
2. 共享栈是否为空
```c
// 判断共享栈是否为空
int isEmpty(SharedStack *s, int stackNum) {
if (stackNum == 1) {
return s->top1 == -1;
} else {
return s->top2 == MAXSIZE;
}
}
```
3. 共享栈是否已满
```c
// 判断共享栈是否已满
int isFull(SharedStack *s) {
return s->top1 + 1 == s->top2;
}
```
4. 共享栈的入栈操作
```c
// 共享栈的入栈操作
int push(SharedStack *s, int stackNum, int x) {
if (isFull(s)) {
return 0; // 栈已满,无法入栈
}
if (stackNum == 1) {
s->data[++s->top1] = x;
} else {
s->data[--s->top2] = x;
}
return 1; // 入栈成功
}
```
5. 共享栈的出栈操作
```c
// 共享栈的出栈操作
int pop(SharedStack *s, int stackNum, int *x) {
if (isEmpty(s, stackNum)) {
return 0; // 栈为空,无法出栈
}
if (stackNum == 1) {
*x = s->data[s->top1--];
} else {
*x = s->data[s->top2++];
}
return 1; // 出栈成功
}
```
6. 共享栈的打印操作
```c
// 共享栈的打印操作
void print(SharedStack *s, int stackNum) {
if (isEmpty(s, stackNum)) {
printf("The stack is empty.\n");
return;
}
if (stackNum == 1) {
printf("Stack 1: ");
for (int i = s->top1; i >= 0; i--) {
printf("%d ", s->data[i]);
}
printf("\n");
} else {
printf("Stack 2: ");
for (int i = s->top2; i < MAXSIZE; i++) {
printf("%d ", s->data[i]);
}
printf("\n");
}
}
```
注意:在使用共享栈进行入栈和出栈操作时,需要指定是操作哪个栈(1或2)。同时,在使用共享栈进行打印操作时,需要指定是打印哪个栈(1或2)。
相关推荐
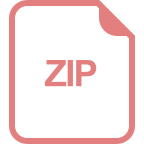
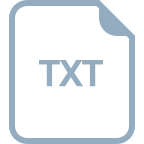














