使用tensorflow实现VGG-19
时间: 2024-05-25 16:05:17 浏览: 13
可以使用TensorFlow中提供的预训练模型来实现VGG-19网络。以下是一些步骤:
1.导入TensorFlow和其他必要的库。
2.下载VGG-19模型的预训练权重文件。这些文件可以在TensorFlow官方提供的链接中找到。
3.建立VGG-19模型的图表,并将模型加载到会话中。
4.准备输入图像并将其传递给模型以进行预测。
5.获取模型输出,这将是一个具有1000个元素的向量,每个元素表示一个类别。
6.使用softmax函数将输出向量转换为概率分布,以便可以识别最可能的类别。
7.根据预测结果,可以采取不同的行动,比如输出类别标签或对图像进行进一步的处理。
以下是一个用TensorFlow实现VGG-19模型的参考代码:
```
import tensorflow as tf
import numpy as np
import urllib.request
def download(url, filename):
urllib.request.urlretrieve(url, filename)
def load_image(filename):
image = tf.keras.preprocessing.image.load_img(filename, target_size=(224, 224))
image = tf.keras.preprocessing.image.img_to_array(image)
image = np.expand_dims(image, axis=0)
image = tf.keras.applications.vgg19.preprocess_input(image)
return image
def VGG19(input_shape=(224,224,3), output_shape=1000, weights_path=None):
vgg19 = tf.keras.applications.VGG19(include_top=True, input_shape=input_shape, weights=None, classes=output_shape)
if weights_path:
vgg19.load_weights(weights_path)
return vgg19
download("http://www.vlfeat.org/matconvnet/models/beta16/imagenet-vgg-verydeep-19.mat", "vgg19_weights.h5")
input_shape = (224, 224, 3)
output_shape = 1000
weights_path = "vgg19_weights.h5"
image = load_image("test_image.jpg")
model = VGG19(input_shape, output_shape, weights_path)
outputs = model.predict(image)
preds = tf.keras.applications.vgg19.decode_predictions(outputs, top=1)[0]
print("Predicted class: {}".format(preds[0][1]))
```
相关推荐
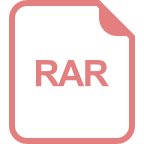
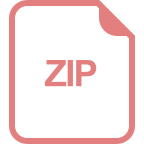














