fastapi type Schedule add delete select update restapi
时间: 2024-05-15 12:19:43 浏览: 17
FastAPI is a modern, fast (high-performance) web framework for building APIs with Python 3.6+ based on standard Python type hints. It provides an easy-to-use interface for creating RESTful APIs with minimal boilerplate code.
To create a RESTful API for a Schedule, you would typically define the following endpoints:
1. Add: HTTP POST request to add a new schedule item.
2. Delete: HTTP DELETE request to delete an existing schedule item.
3. Select: HTTP GET request to retrieve a list of all schedule items or a single schedule item by ID.
4. Update: HTTP PUT request to update an existing schedule item.
Here is an example implementation of these endpoints using FastAPI:
```python
from typing import List
from fastapi import FastAPI
app = FastAPI()
class Schedule:
def __init__(self, id: int, title: str, description: str, date: str):
self.id = id
self.title = title
self.description = description
self.date = date
# Mock data
schedule_items = [
Schedule(1, "Meeting", "Weekly team meeting", "2022-01-05T10:00:00"),
Schedule(2, "Lunch", "Lunch with clients", "2022-01-06T12:30:00"),
Schedule(3, "Conference", "Python conference", "2022-01-10T09:00:00"),
]
@app.post("/schedule")
def add_schedule_item(schedule: Schedule):
"""
Add a new schedule item.
"""
schedule_items.append(schedule)
return {"message": "Schedule item added successfully."}
@app.delete("/schedule/{schedule_id}")
def delete_schedule_item(schedule_id: int):
"""
Delete an existing schedule item by ID.
"""
for i, schedule in enumerate(schedule_items):
if schedule.id == schedule_id:
del schedule_items[i]
return {"message": "Schedule item deleted successfully."}
return {"error": "Schedule item not found."}
@app.get("/schedule")
def get_all_schedule_items() -> List[Schedule]:
"""
Retrieve a list of all schedule items.
"""
return schedule_items
@app.get("/schedule/{schedule_id}")
def get_schedule_item(schedule_id: int) -> Schedule:
"""
Retrieve a single schedule item by ID.
"""
for schedule in schedule_items:
if schedule.id == schedule_id:
return schedule
return {"error": "Schedule item not found."}
@app.put("/schedule/{schedule_id}")
def update_schedule_item(schedule_id: int, schedule: Schedule):
"""
Update an existing schedule item by ID.
"""
for i, s in enumerate(schedule_items):
if s.id == schedule_id:
schedule_items[i] = schedule
return {"message": "Schedule item updated successfully."}
return {"error": "Schedule item not found."}
```
With this implementation, you can use HTTP requests to add, delete, select, and update schedule items via the API. For example, to add a new schedule item, you could send a POST request to http://localhost:8000/schedule with a JSON payload containing the schedule data:
```json
{
"id": 4,
"title": "Training",
"description": "Training session for new recruits",
"date": "2022-01-15T14:00:00"
}
```
You could then use GET requests to retrieve the list of all schedule items or a single schedule item by ID, and PUT and DELETE requests to update or delete existing schedule items.
相关推荐
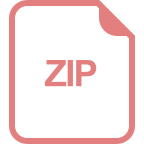
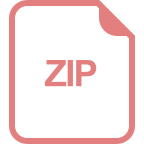
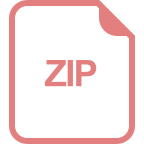














