md5加密hashmap
时间: 2024-01-12 14:22:28 浏览: 85
对于MD5加密和HashMap,这两个概念是不相关的。MD5是一种常用的哈希算法,用于将任意长度的数据转换为固定长度的哈希值。而HashMap是Java中的一种数据结构,用于存储键值对。
如果你想要使用MD5对HashMap中的值进行加密,你需要遍历HashMap中的每个值,将其转换为字符串,然后使用MD5算法对字符串进行加密。下面是一个示例代码:
```java
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
// 创建一个HashMap
Map<String, String> hashMap = new HashMap<>();
hashMap.put("key1", "value1");
hashMap.put("key2", "value2");
hashMap.put("key3", "value3");
// 对HashMap中的值进行MD5加密
Map<String, String> encryptedHashMap = new HashMap<>();
try {
MessageDigest md = MessageDigest.getInstance("MD5");
for (Map.Entry<String, String> entry : hashMap.entrySet()) {
String key = entry.getKey();
String value = entry.getValue();
byte[] valueBytes = value.getBytes();
byte[] encryptedBytes = md.digest(valueBytes);
StringBuilder sb = new StringBuilder();
for (byte b : encryptedBytes) {
sb.append(String.format("%02x", b));
}
String encryptedValue = sb.toString();
encryptedHashMap.put(key, encryptedValue);
}
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
// 打印加密后的HashMap
for (Map.Entry<String, String> entry : encryptedHashMap.entrySet()) {
System.out.println(entry.getKey() + ": " + entry.getValue());
}
}
}
```
这段代码首先创建了一个HashMap,并向其中添加了一些键值对。然后,使用MD5算法对HashMap中的值进行加密,并将加密后的值存储在另一个HashMap中。最后,打印加密后的HashMap。
阅读全文
相关推荐



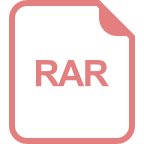












