java httpclient 接口_Java利用httpclient通过get、post方式调用https接口的方法
时间: 2023-11-28 21:04:35 浏览: 146
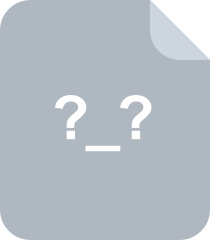
java通过HTTP调用接口(Get请求和Post请求)
Java中可以使用Apache HttpClient库来发送HTTP请求,包括GET、POST等方式,也可以通过HttpClient库发送HTTPS请求。使用HttpClient发送HTTPS请求需要添加SSL证书和设置SSL连接的相关参数。
以下是通过HttpClient库发送GET、POST请求的示例代码:
1. 发送GET请求
```java
import org.apache.http.client.methods.HttpGet;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class HttpClientTest {
public static void main(String[] args) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
String url = "http://www.example.com/api?param1=value1¶m2=value2";
HttpGet httpGet = new HttpGet(url);
String response = null;
try (CloseableHttpResponse httpResponse = httpClient.execute(httpGet)) {
response = EntityUtils.toString(httpResponse.getEntity());
}
System.out.println(response);
}
}
```
2. 发送POST请求
```java
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
public class HttpClientTest {
public static void main(String[] args) throws Exception {
CloseableHttpClient httpClient = HttpClients.createDefault();
String url = "http://www.example.com/api";
HttpPost httpPost = new HttpPost(url);
httpPost.setHeader("Content-Type", "application/json;charset=UTF-8");
String requestBody = "{\"param1\":\"value1\",\"param2\":\"value2\"}";
httpPost.setEntity(new StringEntity(requestBody, "UTF-8"));
String response = null;
try (CloseableHttpResponse httpResponse = httpClient.execute(httpPost)) {
response = EntityUtils.toString(httpResponse.getEntity());
}
System.out.println(response);
}
}
```
如果需要发送HTTPS请求,则需要添加SSL证书和设置SSL连接的相关参数。具体可以参考以下示例代码:
```java
import org.apache.http.client.config.RequestConfig;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.config.Registry;
import org.apache.http.config.RegistryBuilder;
import org.apache.http.conn.socket.ConnectionSocketFactory;
import org.apache.http.conn.socket.PlainConnectionSocketFactory;
import org.apache.http.conn.ssl.SSLConnectionSocketFactory;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.impl.conn.PoolingHttpClientConnectionManager;
import org.apache.http.ssl.SSLContexts;
import org.apache.http.ssl.TrustStrategy;
import org.apache.http.util.EntityUtils;
import javax.net.ssl.HostnameVerifier;
import javax.net.ssl.SSLContext;
import javax.net.ssl.SSLSession;
import java.security.cert.CertificateException;
import java.security.cert.X509Certificate;
public class HttpClientTest {
public static void main(String[] args) throws Exception {
SSLContext sslContext = SSLContexts.custom()
.loadTrustMaterial(null, new TrustStrategy() {
@Override
public boolean isTrusted(X509Certificate[] chain, String authType) throws CertificateException {
return true;
}
}).build();
HostnameVerifier hostnameVerifier = new HostnameVerifier() {
@Override
public boolean verify(String hostname, SSLSession session) {
return true;
}
};
SSLConnectionSocketFactory sslSocketFactory = new SSLConnectionSocketFactory(sslContext, hostnameVerifier);
Registry<ConnectionSocketFactory> socketFactoryRegistry = RegistryBuilder.<ConnectionSocketFactory>create()
.register("https", sslSocketFactory)
.register("http", new PlainConnectionSocketFactory())
.build();
PoolingHttpClientConnectionManager connectionManager = new PoolingHttpClientConnectionManager(socketFactoryRegistry);
connectionManager.setMaxTotal(200);
connectionManager.setDefaultMaxPerRoute(20);
RequestConfig requestConfig = RequestConfig.custom()
.setSocketTimeout(5000)
.setConnectTimeout(5000)
.setConnectionRequestTimeout(5000)
.build();
CloseableHttpClient httpClient = HttpClients.custom()
.setConnectionManager(connectionManager)
.setDefaultRequestConfig(requestConfig)
.build();
String url = "https://www.example.com/api?param1=value1¶m2=value2";
HttpGet httpGet = new HttpGet(url);
String response = null;
try (CloseableHttpResponse httpResponse = httpClient.execute(httpGet)) {
response = EntityUtils.toString(httpResponse.getEntity());
}
System.out.println(response);
}
}
```
需要注意的是,以上代码中的SSL证书验证方式为信任所有证书,不建议在生产环境中使用。建议根据实际情况设置合适的证书验证方式。
阅读全文
相关推荐
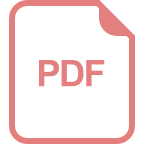
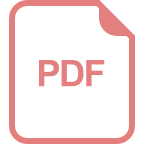
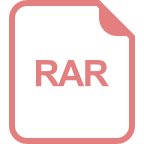


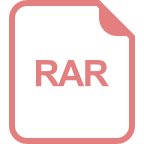
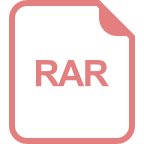
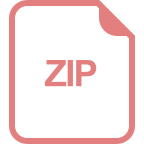
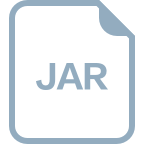
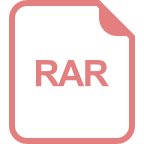
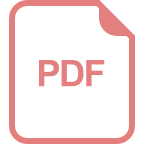
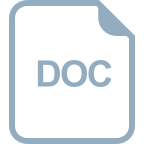
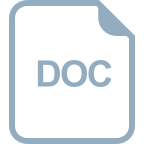
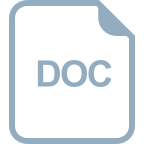
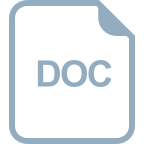
