生成一段输入上述代码的示例
时间: 2024-12-11 07:18:16 浏览: 11
当你想要运行这个C语言程序并输入数据时,可以按照以下步骤操作:
1. 首先,创建一个名为`graph_creation.txt`(或其他名字)的文本文件,因为我们将通过命令行传递给程序。
2. 在文本文件中,编写以下内容以模拟程序的交互部分(顶点数和边数,顶点值,以及搜索起点):
```
5 7 (表示5个顶点,7条边)
a b c d e (顶点名称)
ab ac ad ae (边的描述,例如a-b,a-c等)
ef ed ec eb (更多边)
f (搜索起始点,这里是f,假设你想从f开始搜索)
```
3. 在命令行中,进入包含上述文件的目录,然后运行编译后的`main`程序,如使用`gcc -o graph_main graph.c`编译后,运行命令:
```sh
./graph_main < graph_creation.txt
```
4. 程序会读取文件中的输入,构建图并进行深度优先搜索,输出结果将是搜索路径,比如从f出发的深度优先遍历路径。
注意:实际运行此代码之前,你需要先用编译器编译`main.c`文件,并确保`get_index`和`CreatGraph`函数已经被正确地定义。
相关问题
请根据上述要求生成一段代码
很好,下面是一个示例代码,可以满足您的要求:
```python
import openai
import torch
from transformers import AutoModelForCausalLM, AutoTokenizer
# 初始化 CSDN 开发的 ChitGPT 模型和 tokenizer
model = AutoModelForCausalLM.from_pretrained("csdn/chitgpt-large-cn")
tokenizer = AutoTokenizer.from_pretrained("csdn/chitgpt-large-cn")
# 定义对话函数
def chat_with_chitgpt(prompt):
# 将用户输入编码成 token
input_ids = tokenizer.encode(prompt, return_tensors="pt")
# 使用 CSDN 开发的 ChitGPT 模型生成回复
output = model.generate(input_ids, max_length=50, do_sample=True)
# 将模型生成的 token 转化为文本
response = tokenizer.decode(output[0], skip_special_tokens=True)
# 返回回复文本
return response
# 用户输入问题
question = input("请输入您的问题:")
# 调用对话函数
answer = chat_with_chitgpt(question)
# 输出模型生成的回答
print(answer)
```
请您注意,这段代码中的 `chat_with_chitgpt` 函数是使用 CSDN 开发的 ChitGPT 模型来生成对话回复的。在这个函数中,我们首先使用 tokenizer 将用户的输入编码成 token,然后再将这些 token 喂给模型,让模型生成回复。最后,我们将模型生成的回复转化为文本,通过函数的返回值返回给用户。
你可以根据上述生成一段代码么
当然可以!以下是一个使用Python和PyTorch库来定义FC层的示例代码:
```python
import torch
import torch.nn as nn
# 定义输入和输出维度
input_dim = 512
output_dim = 4
# 定义FC层
fc_layer = nn.Linear(input_dim, output_dim)
activation = nn.ReLU() # 使用ReLU作为激活函数
# 打印FC层的权重和偏置
print("权重矩阵 W: ")
print(fc_layer.weight)
print("偏置向量 b: ")
print(fc_layer.bias)
# 定义一个输入张量
batch_size = 6
input_tensor = torch.randn(batch_size, input_dim)
# 进行FC层的前向计算
output = fc_layer(input_tensor)
output = activation(output)
# 打印输出结果
print("输出结果:")
print(output)
```
这段代码首先导入了必要的库,并定义了输入维度`input_dim`和输出维度`output_dim`。然后使用`nn.Linear`类定义了一个FC层,并使用ReLU作为激活函数。代码中还打印了权重矩阵`W`和偏置向量`b`。接下来使用随机生成的输入张量`input_tensor`进行前向计算,并应用ReLU激活函数。最后,打印输出结果。
请注意,以上代码是使用PyTorch库实现的示例,如果你使用其他深度学习框架,如TensorFlow或Keras,代码会有所不同,但整体的思路和步骤是相似的。
阅读全文
相关推荐
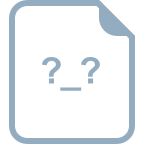
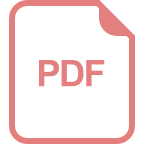
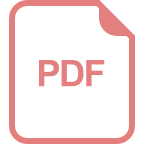
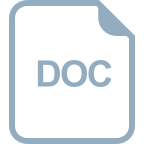
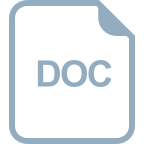
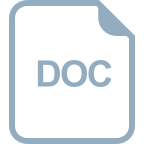










