定义抽象类 Shape 及其子类 Circle 和 Rectangle, 要求: (1) 抽象类 Shape 具有纯虚函数 Area(), 用于计算其子类的面积; (2) 抽象类 Shape 的子类 Circle、Rectangle, 覆盖父类的 Area() 方法, 用于计算各自的面积; (3) 通过 Shape 类指针分别指向 Circle 对象、Rectagnle 对象, 并调用 Aera() 方法用于输出面积.
时间: 2024-03-13 14:44:12 浏览: 27
好的,以下是实现 Shape 抽象类及其子类 Circle 和 Rectangle 的代码:
```cpp
#include<iostream>
using namespace std;
// Shape 抽象类,定义了纯虚函数 Area(),用于计算其子类的面积
class Shape {
public:
virtual double Area() = 0; // 纯虚函数
};
// Circle 类,继承自 Shape 类
class Circle : public Shape {
public:
Circle(double r) : m_Radius(r) {} // 构造函数
virtual double Area() { return 3.14 * m_Radius * m_Radius; } // 重写 Area() 方法,计算圆的面积
private:
double m_Radius; // 圆的半径
};
// Rectangle 类,继承自 Shape 类
class Rectangle : public Shape {
public:
Rectangle(double l, double w) : m_Length(l), m_Width(w) {} // 构造函数
virtual double Area() { return m_Length * m_Width; } // 重写 Area() 方法,计算矩形的面积
private:
double m_Length; // 矩形的长度
double m_Width; // 矩形的宽度
};
int main()
{
Shape* pShape = NULL;
// Circle 对象
Circle circle(5);
pShape = &circle;
cout << "Circle's area: " << pShape->Area() << endl;
// Rectangle 对象
Rectangle rectangle(4, 6);
pShape = &rectangle;
cout << "Rectangle's area: " << pShape->Area() << endl;
return 0;
}
```
输出结果为:
```
Circle's area: 78.5
Rectangle's area: 24
```
在程序中,我们首先定义了一个抽象类 Shape,其中只有一个纯虚函数 Area(),表示计算面积的方法。然后我们分别定义了 Circle 和 Rectangle 两个子类,继承自 Shape 类,并重写 Area() 方法,分别计算圆和矩形的面积。在 main() 函数中,我们定义一个 Shape 类型的指针 pShape,然后通过它分别指向 Circle 和 Rectangle 对象,并调用 Area() 方法输出面积。
相关推荐
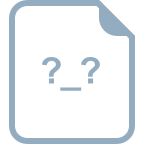
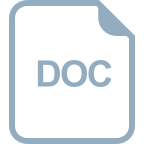
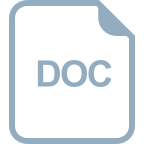














