用Android stdio写一个计步器,写出java代码和xml代码,以及写出权限,及具体步骤
时间: 2024-03-14 17:48:36 浏览: 171
好的,以下是实现一个简单计步器的具体步骤:
1. 首先,在Android Studio中创建一个新的项目,取一个合适的项目名称。
2. 在项目中创建一个新的Activity,用于实现计步器的UI界面。
3. 在Activity对应的XML布局文件中,添加一个TextView用于显示步数,以及一个Button用于重置步数。
4. 在AndroidManifest.xml文件中添加步数统计所需的权限:
```
<uses-permission android:name="android.permission.ACTIVITY_RECOGNITION" />
```
5. 在Activity中添加以下代码,用于实现计步器的逻辑:
```java
public class MainActivity extends AppCompatActivity implements SensorEventListener {
private TextView stepCountTextView;
private Button resetButton;
private SensorManager sensorManager;
private Sensor stepSensor;
private int stepCount;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
stepCountTextView = findViewById(R.id.step_count_text_view);
resetButton = findViewById(R.id.reset_button);
sensorManager = (SensorManager) getSystemService(Context.SENSOR_SERVICE);
if (sensorManager.getDefaultSensor(Sensor.TYPE_STEP_COUNTER) != null) {
stepSensor = sensorManager.getDefaultSensor(Sensor.TYPE_STEP_COUNTER);
} else {
Toast.makeText(this, "Step counter sensor not available", Toast.LENGTH_SHORT).show();
}
resetButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
stepCount = 0;
updateStepCountTextView();
}
});
}
@Override
protected void onResume() {
super.onResume();
if (stepSensor != null) {
sensorManager.registerListener(this, stepSensor, SensorManager.SENSOR_DELAY_NORMAL);
}
}
@Override
protected void onPause() {
super.onPause();
if (stepSensor != null) {
sensorManager.unregisterListener(this);
}
}
@Override
public void onSensorChanged(SensorEvent event) {
if (event.sensor.getType() == Sensor.TYPE_STEP_COUNTER) {
stepCount = (int) event.values[0];
updateStepCountTextView();
}
}
@Override
public void onAccuracyChanged(Sensor sensor, int accuracy) {
// do nothing
}
private void updateStepCountTextView() {
stepCountTextView.setText("Step count: " + stepCount);
}
}
```
6. 最后,运行应用程序并测试计步器是否正常工作。
这里是XML布局文件的代码:
```xml
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:id="@+id/activity_main"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context="com.example.stepcounter.MainActivity">
<TextView
android:id="@+id/step_count_text_view"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Step count: 0"
android:textSize="24sp"
android:textStyle="bold"
android:layout_centerInParent="true"/>
<Button
android:id="@+id/reset_button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Reset"
android:layout_below="@id/step_count_text_view"
android:layout_centerHorizontal="true"
android:layout_marginTop="16dp"/>
</RelativeLayout>
```
希望这能帮到你!
阅读全文
相关推荐
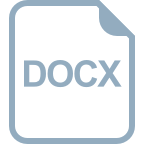
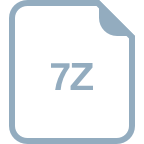
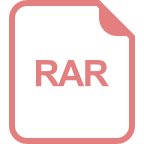















