给定一个单链表 L1→L2→⋯→Ln−1→Ln,请编写程序将链表重新排列为 Ln→L1→Ln−1→L2→⋯。例如:给定L为1→2→3→4→5→6,则输出应该为6→1→5→2→4→3。
时间: 2023-11-18 17:55:13 浏览: 203
给定一个单链表 L1→L2→⋯→Ln−1→Ln,可以按照以下步骤重新排列链表:
1. 找到链表的中间节点,可以使用快慢指针的方法,将链表分为两个部分;
2. 将后半部分链表翻转;
3. 将两个链表合并,交替插入节点即可。
具体实现可以参考以下代码:
```python
class Solution:
def reorderList(self, head: ListNode) -> None:
"""
Do not return anything, modify head in-place instead.
"""
if not head or not head.next:
return
# 找到链表的中间节点
slow, fast = head, head
while fast.next and fast.next.next:
slow = slow.next
fast = fast.next.next
# 将后半部分链表翻转
prev, curr = None, slow.next
slow.next = None
while curr:
next_node = curr.next
curr.next = prev
prev = curr
curr = next_node
# 将两个链表合并,交替插入节点
p1, p2 = head, prev
while p2:
next_node1 = p1.next
next_node2 = p2.next
p1.next = p2
p2.next = next_node1
p1 = next_node1
p2 = next_node2
```
阅读全文
相关推荐
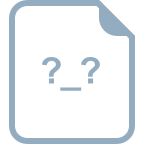
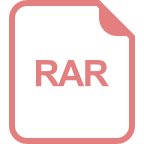
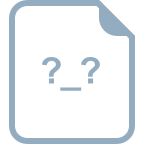







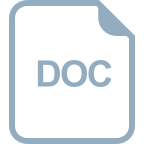




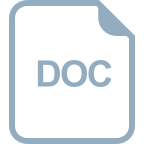