go语言给定一个短字符串(不含空格),再给定若干字符串,在这些字符串中删除所含有的短字符串。
时间: 2023-10-01 19:09:11 浏览: 37
可以使用 strings 包中的 Replace 函数来删除某个字符串中的子串。具体的实现代码如下:
```go
package main
import (
"fmt"
"strings"
)
func main() {
shortStr := "abc"
strList := []string{"abcdefg", "bcd", "efg", "abc", "hijkabc", "ab", "c"}
for i := 0; i < len(strList); i++ {
strList[i] = strings.ReplaceAll(strList[i], shortStr, "")
}
fmt.Println(strList)
}
```
以上代码中,我们首先定义了一个短字符串 `shortStr`,以及一个字符串列表 `strList`。然后使用 for 循环遍历 `strList`,对每个字符串都使用 `strings.ReplaceAll` 函数将其中的 `shortStr` 子串删除。最后输出处理后的字符串列表即可。
需要注意的是,`strings.ReplaceAll` 函数会将所有的 `shortStr` 子串都删除,如果只想删除首次出现的子串,可以使用 `strings.Replace` 函数。
相关问题
python给定一个短字符串(不含空格),再给定若干字符串,在这些字符串中删除所含有的短字符串。
### 回答1:
可以使用 Python 的字符串方法 `replace()` 来实现。例如:
```
short_str = "abc"
strings = ["abcdef", "ghabc", "ijkl"]
for i in range(len(strings)):
strings[i] = strings[i].replace(short_str, "")
print(strings)
# 输出:['def', 'gh', 'ijkl']
```
上面的代码中,首先定义了一个短字符串 `short_str` 和一个字符串列表 `strings`,然后使用循环遍历 `strings` 中的每个字符串,并在每个字符串中使用 `replace()` 方法删除 `short_str`。最后输出修改后的字符串列表。
### 回答2:
可以使用Python的字符串操作方法来解决这个问题。步骤如下:
1. 定义一个短字符串short_str。
2. 定义一个字符串列表str_list,保存若干字符串。
3. 遍历str_list中的每个字符串。
4. 在每次遍历时,使用字符串的replace方法将短字符串short_str替换为空字符串。
5. 将替换后的字符串保存回原来的位置。
代码示例:
```python
short_str = "短字符串"
str_list = ["这是一段包含短字符串的字符串", "这是另一段字符串"]
for i in range(len(str_list)):
str_list[i] = str_list[i].replace(short_str, "")
```
这段代码首先定义了一个短字符串short_str和一个字符串列表str_list。然后使用循环遍历str_list中的每个字符串,在每次遍历时调用replace方法,将短字符串替换为空字符串。最后,替换后的字符串将保存回原来的位置。
运行该代码后,str_list中的每个字符串中都将不再包含短字符串short_str。
### 回答3:
可以使用Python中的字符串操作来解决这个问题。首先,从输入中获取短字符串和若干字符串。
然后,我们可以使用字符串的replace方法来删除所含有的短字符串。这个方法会将原字符串中所有匹配的子字符串替换为指定的字符串(这里我们可以将其替换为空字符串)。
以下是一个示例代码:
```python
# 获取短字符串
short_string = input("请输入一个短字符串(不含空格):")
# 获取若干字符串
strings = input("请输入若干字符串,空格分隔:").split()
# 删除所含有的短字符串
for i in range(len(strings)):
strings[i] = strings[i].replace(short_string, "")
# 输出结果
print("删除短字符串后的字符串:")
for string in strings:
print(string)
```
使用这段代码,当输入短字符串为"abc",若干字符串为"abcdefg"、"abccba"、"hijkabc"时,输出结果将为:
```
删除短字符串后的字符串:
defg
cba
hijk
```
这样,我们就成功删除了这些字符串中所含有的短字符串。
用go语言实现给定一个短字符串(不含空格),再给定若干字符串,在这些字符串中删除所含有的短字符串。 输入格式: 先输入一个指定将被删除的字符串,再输入一串将被删除指定字符串的长字符串。 输出格式: 删除长字符串中所有包含指定短字符串的剩余部分 输入样例: 请输入一串长字符串: This is a test. This is only a test. 请输入指定将被删除的字符串: is 输出样例: 过滤后的字符串: Th a test. Th only a test.
好的,这是一个字符串处理的问题,可以使用 Go 语言的 strings 包来实现。以下是实现的代码示例:
```go
package main
import (
"fmt"
"strings"
)
func main() {
var longStr, shortStr string
fmt.Print("请输入一串长字符串: ")
fmt.Scan(&longStr)
fmt.Print("请输入指定将被删除的字符串: ")
fmt.Scan(&shortStr)
filteredStr := strings.ReplaceAll(longStr, shortStr, "")
fmt.Println("过滤后的字符串:", filteredStr)
}
```
运行程序后,按照提示输入长字符串和要删除的字符串,程序会输出过滤后的字符串。
相关推荐
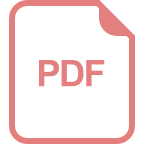
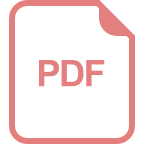
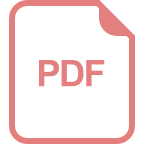
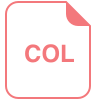
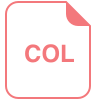
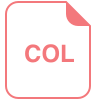
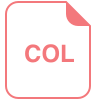
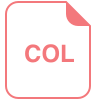







