from sklearn.model_selection import StratifiedKFold kfold = StratifiedKFold(n_splits=5, shuffle=True, random_state=42) for train, test in kfold.split(X, y): model = create_model() model.fit(X[train], y[train], validation_data=(X[test], y[test]), epochs=10, batch_size=32)这段代码中的训练集与测试集可以为3:7开吗?
时间: 2024-03-07 20:51:25 浏览: 22
可以的。在这段代码中,使用StratifiedKFold函数进行了5折交叉验证,每次将数据集分成训练集和测试集。数据集的划分比例取决于你传入的参数。在这里,数据集被随机shuffle过,因此训练集和测试集的比例可能不是完全相同的。你可以根据具体情况调整参数,比如把n_splits设置为1,这样就只会有一组划分,你可以将其划分成3:7的训练集和测试集。
相关问题
import pandas as pd import numpy as np from sklearn.model_selection import StratifiedKFold, KFold from sklearn.svm import SVR train = pd.read_csv('train.csv') test = pd.read_csv('test.csv') # 分离数据集 X_train_c = train.drop(['ID', 'CLASS'], axis=1).values y_train_c = train['CLASS'].values X_test_c = test.drop(['ID'], axis=1).values nfold = 5 kf = KFold(n_splits=nfold, shuffle=True, random_state=2020) prediction1 = np.zeros((len(X_test_c),)) i = 0 for train_index, valid_index in kf.split(X_train_c, y_train_c): print("\nFold {}".format(i + 1)) X_train, label_train = X_train_c[train_index], y_train_c[train_index] X_valid, label_valid = X_train_c[valid_index], y_train_c[valid_index] clf = SVR(kernel='rbf', C=1, gamma='scale') clf.fit(X_train, label_train) x1 = clf.predict(X_valid) y1 = clf.predict(X_test_c) prediction1 += y1 / nfold i += 1 result1 = np.round(prediction1) id_ = range(210, 314) df = pd.DataFrame({'ID': id_, 'CLASS': result1}) df.to_csv("baseline.csv", index=False)
这段代码是一个基线模型,使用了支持向量回归(SVR)算法,通过 K 折交叉验证来训练模型,并对测试集进行预测。其中,训练数据集和测试数据集均为 CSV 格式的文件,需要使用 pandas 库读取。具体的实现过程如下:
1. 读取训练集和测试集的数据,并进行预处理。
```python
train = pd.read_csv('train.csv')
test = pd.read_csv('test.csv')
X_train_c = train.drop(['ID', 'CLASS'], axis=1).values
y_train_c = train['CLASS'].values
X_test_c = test.drop(['ID'], axis=1).values
```
2. 定义 K 折交叉验证器,并进行模型训练和预测。
```python
nfold = 5
kf = KFold(n_splits=nfold, shuffle=True, random_state=2020)
prediction1 = np.zeros((len(X_test_c),))
i = 0
for train_index, valid_index in kf.split(X_train_c, y_train_c):
print("\nFold {}".format(i + 1))
X_train, label_train = X_train_c[train_index], y_train_c[train_index]
X_valid, label_valid = X_train_c[valid_index], y_train_c[valid_index]
clf = SVR(kernel='rbf', C=1, gamma='scale')
clf.fit(X_train, label_train)
x1 = clf.predict(X_valid)
y1 = clf.predict(X_test_c)
prediction1 += y1 / nfold
i += 1
```
3. 对测试集的预测结果进行处理,并将结果保存到 CSV 文件中。
```python
result1 = np.round(prediction1)
id_ = range(210, 314)
df = pd.DataFrame({'ID': id_, 'CLASS': result1})
df.to_csv("baseline.csv", index=False)
```
其中,`prediction1` 是对测试集的预测结果进行累加的数组,`result1` 是将累加结果四舍五入后得到的最终预测结果。最后将结果保存到 CSV 文件中,方便后续的提交。
逐行解释下面的代码:from sklearn.datasets import load_breast_cancer from sklearn.model_selection import train_test_split, GridSearchCV, KFold from sklearn.ensemble import RandomForestClassifier data = load_breast_cancer() X_train, X_test, y_train, y_test = train_test_split(data.data, data.target, test_size=0.3, random_state=42) kf = KFold(n_splits=5, shuffle=True, random_state=42) param_grid = {'n_estimators': range(1, 21, 1), 'max_depth': range(5, 16)} rf = RandomForestClassifier(random_state=42) grid_search = GridSearchCV(rf, param_grid=param_grid, cv=kf, n_jobs=-1) grid_search.fit(X_train, y_train) best_rf = RandomForestClassifier(n_estimators=grid_search.best_params_['n_estimators'], max_depth=grid_search.best_params_['max_depth'], random_state=42) best_rf.fit(X_train, y_train) y_pred = best_rf.predict(X_test)
这段代码的作用是使用随机森林算法对乳腺癌数据集进行分类,并找到最佳的模型参数。
首先,代码从sklearn.datasets库中导入了load_breast_cancer函数和从sklearn.model_selection库中导入了train_test_split、GridSearchCV和KFold函数以及从sklearn.ensemble库中导入了RandomForestClassifier类。
然后,代码调用load_breast_cancer()函数来加载乳腺癌数据集。接着,使用train_test_split函数将数据集分成训练集和测试集。其中,test_size参数指定测试集所占比例为30%,random_state参数用于设置随机数种子,以确保每次运行代码时得到的结果相同。
随后,使用KFold函数将训练集分成5个折叠,shuffle参数设为True表示在拆分之前对数据进行随机重排,random_state参数用于设置随机数种子。
接下来,定义一个字典param_grid,其中包含了随机森林算法的两个参数:n_estimators和max_depth。n_estimators参数表示随机森林中决策树的数量,max_depth参数表示每个决策树的最大深度。param_grid的取值范围分别为1到20和5到15。
然后,创建一个RandomForestClassifier类的实例rf,将其作为参数传递给GridSearchCV函数,用于在给定的参数空间中搜索最佳的参数组合。cv参数指定使用的交叉验证策略,n_jobs参数指定使用的CPU数量。
接着,调用fit方法来训练模型并搜索最佳参数组合,将结果存储在grid_search对象中。
接下来,创建一个新的RandomForestClassifier类的实例best_rf,使用grid_search.best_params_字典中的最佳参数组合来初始化该实例,并将其用于训练数据。最后,使用best_rf.predict方法对测试数据进行预测,将结果存储在y_pred变量中。
相关推荐
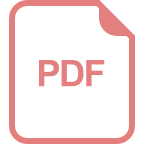












