C# c#键值对异步
时间: 2023-07-12 14:18:38 浏览: 129
在C#中,可以使用异步方法来处理键值对。具体实现可以使用异步的Dictionary类,它提供了一些异步的方法来访问和操作键值对。例如,可以使用异步的AddAsync方法来向字典中添加键值对,使用异步的ContainsKeyAsync方法来检查是否存在某个键等等。以下是一个使用异步方法处理键值对的示例代码:
```csharp
using System.Collections.Generic;
using System.Threading.Tasks;
class Program
{
static Dictionary<string, string> dictionary = new Dictionary<string, string>();
static async Task Main(string[] args)
{
await dictionary.AddAsync("key1", "value1");
bool containsKey = await dictionary.ContainsKeyAsync("key1");
string value = await dictionary.GetValueAsync("key1");
await dictionary.RemoveAsync("key1");
}
}
public static class DictionaryExtensions
{
public static async Task AddAsync<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, TKey key, TValue value)
{
await Task.Run(() => dictionary.Add(key, value));
}
public static async Task<bool> ContainsKeyAsync<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, TKey key)
{
return await Task.Run(() => dictionary.ContainsKey(key));
}
public static async Task<TValue> GetValueAsync<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, TKey key)
{
return await Task.Run(() => dictionary[key]);
}
public static async Task RemoveAsync<TKey, TValue>(this Dictionary<TKey, TValue> dictionary, TKey key)
{
await Task.Run(() => dictionary.Remove(key));
}
}
```
在这个示例中,我们使用了异步方法AddAsync、ContainsKeyAsync、GetValueAsync和RemoveAsync来向字典中添加、检查、获取和删除键值对。这些方法都是异步的,可以在异步环境下使用,比如在异步方法或线程池任务中。
阅读全文
相关推荐
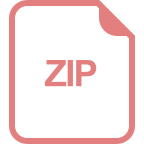
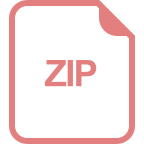
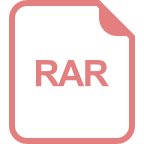
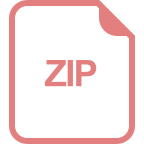
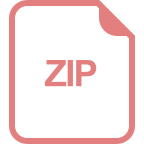
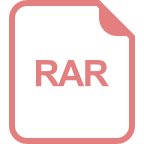
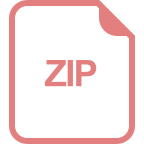
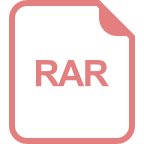
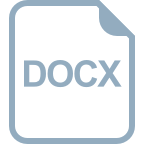
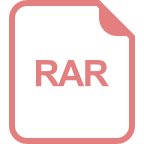
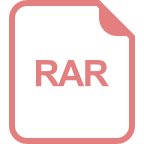
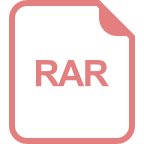
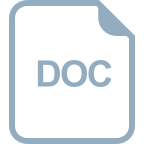
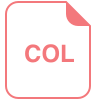
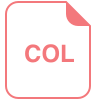