电子邮件加密项目代码
时间: 2023-08-26 13:05:27 浏览: 49
这里提供一个使用Python实现的电子邮件加密项目代码,使用了PGP (Pretty Good Privacy)算法进行加密和解密。
```python
import gnupg
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.application import MIMEApplication
class EmailSender:
def __init__(self, gpg_passphrase):
self.gpg = gnupg.GPG()
self.gpg.passphrase = gpg_passphrase
def _encrypt(self, message, recipient_key):
encrypted_data = self.gpg.encrypt(message, recipient_key, always_trust=True)
return str(encrypted_data)
def _send_email(self, sender_email, sender_password, recipient_email, subject, message, attachment=None):
msg = MIMEMultipart()
msg['From'] = sender_email
msg['To'] = recipient_email
msg['Subject'] = subject
if attachment:
with open(attachment, "rb") as f:
data = f.read()
part = MIMEApplication(data, Name=attachment)
part['Content-Disposition'] = 'attachment; filename="%s"' % attachment
msg.attach(part)
msg.attach(MIMEText(message, 'plain'))
server = smtplib.SMTP('smtp.gmail.com', 587)
server.starttls()
server.login(sender_email, sender_password)
server.sendmail(sender_email, recipient_email, msg.as_string())
server.quit()
def send_encrypted_email(self, sender_email, sender_password, recipient_email, subject, message, recipient_key, attachment=None):
encrypted_message = self._encrypt(message, recipient_key)
self._send_email(sender_email, sender_password, recipient_email, subject, encrypted_message, attachment)
```
使用方法:
```python
# 初始化 EmailSender
sender = EmailSender(gpg_passphrase='your_gpg_passphrase')
# 发送加密邮件
sender.send_encrypted_email(
sender_email='your_sender_email@gmail.com',
sender_password='your_sender_email_password',
recipient_email='recipient_email@gmail.com',
subject='Test email',
message='This is a test email.',
recipient_key='recipient_public_key',
attachment='path/to/attachment'
)
```
请注意:在使用本代码之前,需要安装 gnupg 和 python-gnupg 模块,并且需要先生成密钥对并导入收件人的公钥。
相关推荐
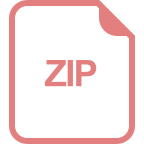
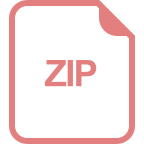
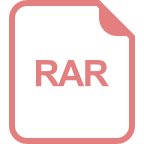














