c语言实现idea算法
时间: 2023-11-20 22:05:15 浏览: 233
IDEA算法是一种广泛使用的加密算法,下面是c语言实现IDEA算法的步骤:
1. 定义IDEA算法所需的数据类型,包括uint16、uint32、byte等。
2. 定义IDEA算法所需的常量,包括密钥长度、轮数、S盒等。
3. 实现IDEA算法所需的函数,包括加密函数、解密函数、密钥扩展函数等。
4. 在主函数中调用IDEA算法函数,实现加密或解密操作。
下面是一个简单的c语言实现IDEA算法的例子:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
typedef unsigned short uint16;
typedef unsigned int uint32;
typedef unsigned char byte;
#define IDEA_KEYLEN 16
#define IDEA_ROUNDS 8
#define IDEA_BLOCKLEN 8
static const uint16 S[8][256] = {...}; // S盒
static const uint16 mul_inv = 0x10001; // 乘法逆元
void idea_expand_key(const byte *key, uint16 *ekey) {
// 密钥扩展函数
...
}
void idea_encrypt_block(const byte *in, byte *out, const uint16 *ekey) {
// 加密函数
...
}
void idea_decrypt_block(const byte *in, byte *out, const uint16 *ekey) {
// 解密函数
...
}
int main() {
byte in[IDEA_BLOCKLEN] = {...}; // 待加密的数据
byte out[IDEA_BLOCKLEN]; // 加密后的数据
byte dec[IDEA_BLOCKLEN]; // 解密后的数据
byte key[IDEA_KEYLEN] = {...}; // 密钥
uint16 ekey[IDEA_ROUNDS*6+4]; // 扩展后的密钥
idea_expand_key(key, ekey); // 扩展密钥
idea_encrypt_block(in, out, ekey); // 加密
idea_decrypt_block(out, dec, ekey); // 解密
return 0;
}
```
阅读全文
相关推荐
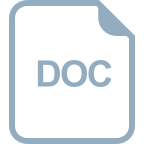
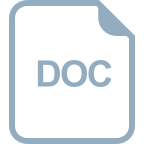
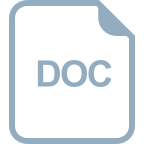
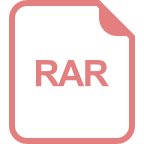
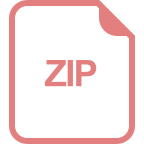
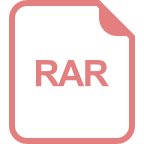
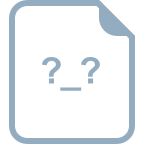
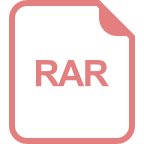
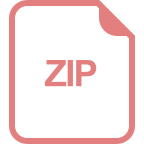
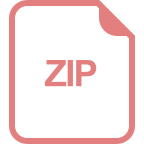
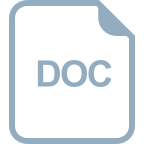
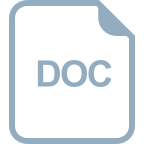
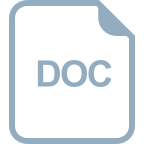


