密码学idea c语言
时间: 2023-11-13 09:53:01 浏览: 43
密码学中的 IDEA(International Data Encryption Algorithm)是一种对称加密算法,它最初由 Xuejia Lai 和 James L. Massey 在1991年提出。它使用了一个128位的密钥和64位的数据块,通过一系列的轮函数对数据进行加密和解密。
以下是一个简单的使用 C 语言实现 IDEA 加密算法的示例:
```c
#include <stdio.h>
#include <stdint.h>
typedef uint16_t WORD;
typedef uint64_t DWORD;
#define IDEA_KEY_SIZE 16
void ideaEncrypt(const WORD *key, const WORD *plainText, WORD *cipherText) {
// 实现 IDEA 加密算法的逻辑
// ...
}
void ideaDecrypt(const WORD *key, const WORD *cipherText, WORD *plainText) {
// 实现 IDEA 解密算法的逻辑
// ...
}
int main() {
// IDEA 密钥
WORD key[IDEA_KEY_SIZE] = {0x1234,0x5678, 0x9abc, 0xdef0,
0x2345, 0x6789, 0xabcde, 0xf012,
0x3456, 0x789a, 0xbcde, 0xf012,
0x4567, 0x89ab, 0xcdef, 0x0123};
// 明文数据
WORD plainText[2] = {0x0123, 0x4567};
// 加密
WORD cipherText[2];
ideaEncrypt(key, plainText, cipherText);
// 解密
WORD decryptedText[2];
ideaDecrypt(key, cipherText, decryptedText);
// 输出结果
printf("Cipher Text: %04X %04X\n", cipherText[0], cipherText[1]);
printf("Decrypted Text: %04X %04X\n", decryptedText[0], decryptedText[1]);
return 0;
}
```
注意:以上代码只是 IDEA 算法的一个简单示例,实际使用中还需要进行更多的安全性和效率优化。
相关推荐
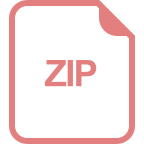
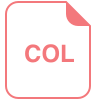
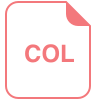
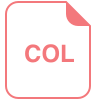
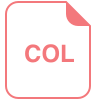
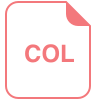









