C++ 读取bmp图片颜色信息
时间: 2023-06-20 18:06:08 浏览: 110
你可以使用C++的标准库和一些第三方库来读取bmp图片的颜色信息。
以下是使用标准库的代码示例:
```c++
#include <iostream>
#include <fstream>
#include <cstdint>
#pragma pack(push, 1)
struct BMPHeader {
char id[2];
uint32_t size;
uint16_t reserved1;
uint16_t reserved2;
uint32_t offset;
uint32_t dib_size;
int32_t width;
int32_t height;
uint16_t planes;
uint16_t bpp;
uint32_t compression;
uint32_t image_size;
int32_t x_ppm;
int32_t y_ppm;
uint32_t color_used;
uint32_t color_important;
};
#pragma pack(pop)
int main() {
std::ifstream file("image.bmp", std::ios::binary); // 打开bmp文件
if (!file.is_open()) {
std::cerr << "Failed to open file\n";
return 1;
}
BMPHeader header;
file.read(reinterpret_cast<char*>(&header), sizeof(header)); // 读取bmp文件头
if (header.id[0] != 'B' || header.id[1] != 'M') {
std::cerr << "Invalid file format\n";
return 1;
}
if (header.bpp != 24) { // 只处理24位bmp图像
std::cerr << "Only 24-bit BMP images are supported\n";
return 1;
}
const int32_t width = header.width;
const int32_t height = std::abs(header.height);
const uint32_t row_size = ((width * 3) + 3) / 4 * 4; // 每行字节数
const uint32_t data_size = row_size * height; // 图像数据大小
std::unique_ptr<uint8_t[]> data(new uint8_t[data_size]); // 分配内存用于存储图像数据
file.read(reinterpret_cast<char*>(data.get()), data_size); // 读取图像数据
// 从图像数据中提取颜色信息
for (int32_t y = 0; y < height; ++y) {
for (int32_t x = 0; x < width; ++x) {
const uint8_t* pixel = &data[y * row_size + x * 3];
const uint8_t red = pixel[2];
const uint8_t green = pixel[1];
const uint8_t blue = pixel[0];
// 在这里处理颜色信息,比如输出每个像素点的RGB值
std::cout << "Pixel (" << x << ", " << y << "): RGB("
<< static_cast<int>(red) << ", "
<< static_cast<int>(green) << ", "
<< static_cast<int>(blue) << ")\n";
}
}
return 0;
}
```
如果你想使用第三方库,可以考虑使用OpenCV库。以下是使用OpenCV读取bmp文件的代码示例:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
int main() {
cv::Mat image = cv::imread("image.bmp", cv::IMREAD_COLOR); // 读取bmp文件
if (image.empty()) {
std::cerr << "Failed to open file\n";
return 1;
}
// 提取颜色信息
for (int32_t y = 0; y < image.rows; ++y) {
for (int32_t x = 0; x < image.cols; ++x) {
const cv::Vec3b& pixel = image.at<cv::Vec3b>(y, x);
const uint8_t red = pixel[2];
const uint8_t green = pixel[1];
const uint8_t blue = pixel[0];
// 在这里处理颜色信息,比如输出每个像素点的RGB值
std::cout << "Pixel (" << x << ", " << y << "): RGB("
<< static_cast<int>(red) << ", "
<< static_cast<int>(green) << ", "
<< static_cast<int>(blue) << ")\n";
}
}
return 0;
}
```
阅读全文
相关推荐








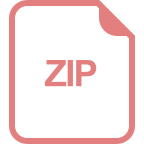







