vue父组件调用子组件的方法,子组件发送消息给父组后
时间: 2024-12-27 15:18:20 浏览: 4
### 实现父组件调用子组件方法
在 Vue 中,为了使父组件能够调用子组件中的特定功能,在子组件内需先定义好待暴露的方法。当采用组合式API时,可在`setup()`函数里利用`expose`选项指定哪些属性或方法可被外界访问[^3]。
对于模板语法编写场景下,则不需要额外声明 expose,默认所有 methods 下的方法都可以被父级通过 ref 访问到[^1]。
#### 父组件代码片段
```html
<template>
<div class="parent">
<!-- 使用ref绑定子组件 -->
<ChildComponent ref="childRef"/>
<button @click="callChildMethod">Call Child Method</button>
</div>
</template>
<script setup>
import { ref } from 'vue'
import ChildComponent from './components/ChildComponent.vue'
const childRef = ref(null)
function callChildMethod() {
if (childRef.value && typeof childRef.value.childFunction === 'function') {
childRef.value.childFunction()
}
}
</script>
```
#### 子组件代码片段
```javascript
// 如果是在 script setup 的情况下
<script setup>
defineExpose({
childFunction: () => console.log('This is a method exposed to parent')
})
</script>
<!-- 或者传统写法 -->
<script>
export default {
name: "ChildComponent",
methods:{
childFunction(){
console.log('This is a method exposed to parent');
}
},
};
</script>
```
### 子组件向父组件发送消息的最佳实践
为了让子组件能通知其父组件某些事件的发生,通常的做法是让子组件触发自定义事件,而这些事件可以在父组件中监听并作出响应。这可以通过 `$emit` 方法完成,它允许子组件发出带有名称的事件,并可以选择附带数据一起传递给监听该事件的父组件处理逻辑[^4]。
#### 修改后的子组件部分
```html
<button @click="$emit('customEvent', message)">Emit Event To Parent</button>
```
此时 `message` 是想要传输至父端的信息变量名;而在父方则应设置相应的侦听器:
#### 对应更新过的父组件部分
```html
<template>
<div class="parent">
<ChildComponent @customEvent="(msg) => handleCustomEvent(msg)" />
</div>
</template>
<script setup>
import ChildComponent from './components/ChildComponent.vue';
function handleCustomEvent(message){
alert(`Received Message From Child Component:${message}`);
}
</script>
```
阅读全文
相关推荐
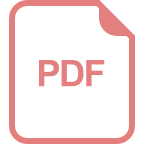
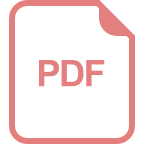
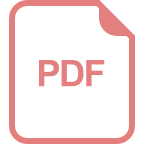
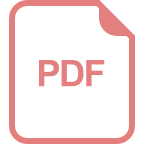
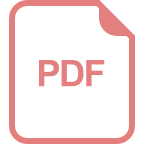
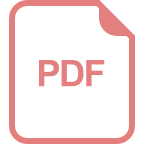
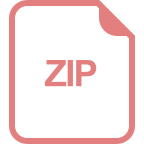
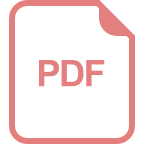
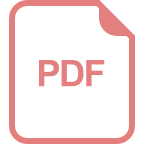
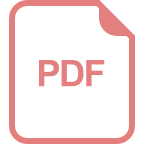
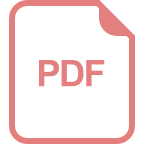
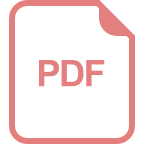
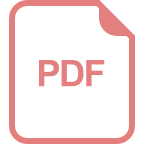
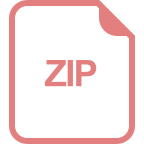
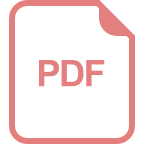
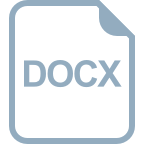
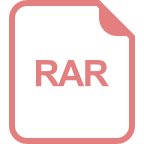
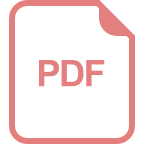