python点云配准算法代码
时间: 2024-01-19 14:18:47 浏览: 192
以下是使用CPD算法实现点云配准的Python代码示例:
```python
import numpy as np
from scipy.spatial import KDTree
from scipy.linalg import orthogonal_procrustes
def cpd_registration(source_points, target_points, max_iterations=50, tolerance=1e-5):
# 初始化变量
num_points = source_points.shape[0]
dim = source_points.shape[1]
R = np.eye(dim)
t = np.zeros((dim, 1))
sigma2 = 1
# 构建KD树
target_tree = KDTree(target_points)
for iteration in range(max_iterations):
# 计算对应点对
correspondences = find_correspondences(source_points, target_tree)
# 计算权重矩阵
W = compute_weight_matrix(correspondences, sigma2)
# 计算对齐后的源点云
aligned_points = align_points(source_points, W, correspondences)
# 计算旋转矩阵和平移向量
R_new, t_new = estimate_transformation(aligned_points, target_points)
# 更新旋转矩阵和平移向量
source_points = np.dot(source_points, R_new.T) + t_new.T
# 计算误差
error = np.linalg.norm(R_new - R) + np.linalg.norm(t_new - t)
# 更新旋转矩阵、平移向量和误差
R = R_new
t = t_new
# 判断是否收敛
if error < tolerance:
break
return R, t
def find_correspondences(source_points, target_tree):
_, indices = target_tree.query(source_points)
return indices
def compute_weight_matrix(correspondences, sigma2):
num_points = correspondences.shape[0]
W = np.zeros((num_points, num_points))
for i in range(num_points):
for j in range(num_points):
diff = correspondences[i] - correspondences[j]
W[i, j] = np.exp(-np.linalg.norm(diff) / (2 * sigma2))
return W
def align_points(source_points, W, correspondences):
num_points = source_points.shape[0]
dim = source_points.shape[1]
aligned_points = np.zeros((num_points, dim))
for i in range(num_points):
aligned_points[i] = np.dot(W[i], source_points[correspondences[i]]) / np.sum(W[i])
return aligned_points
def estimate_transformation(source_points, target_points):
R, _ = orthogonal_procrustes(source_points, target_points)
t = np.mean(target_points, axis=0) - np.mean(source_points, axis=0)
return R, t
# 示例用法
source_points = np.array([[1, 2], [3, 4], [5,6]])
target_points = np.array([[2, 3], [4, 5], [6, 7]])
R, t = cpd_registration(source_points, target_points)
print("旋转矩阵:")
print(R)
print("平移向量:")
print(t)
```
阅读全文
相关推荐

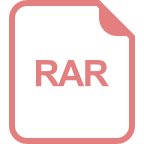
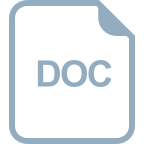


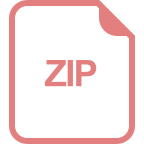
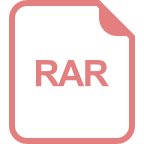
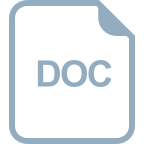







