python实现icp点云配准代码
时间: 2024-07-16 08:00:51 浏览: 123
ICP (Iterative Closest Point) 算法是一种常用的点云配准方法,在Python中,我们可以利用开源库如`scikit-image`或`pybind11`结合一些自定义函数来实现基本的ICP算法。这里提供一个简化版的代码示例:
```python
import numpy as np
from scipy.spatial.distance import cdist
from sklearn.metrics.pairwise import euclidean_distances
# 假设我们有两个点云(列表形式,每个元素是一个点的二维坐标)
def icp(point_cloud_a, point_cloud_b, init_transform=None, max_iterations=100, tolerance=1e-6):
if not init_transform:
# 初始化位姿估计,比如随机旋转和平移
transform = np.eye(4)
else:
transform = init_transform.copy()
for _ in range(max_iterations):
# 将A经过当前变换转换到B的空间
transformed_point_cloud_a = np.dot(transform[:3, :3], point_cloud_a.T).T + transform[:3, 3]
# 计算对应点的距离
distances = euclidean_distances(transformed_point_cloud_a, point_cloud_b)
# 找出最近的匹配对
closest_indices = np.argmin(distances, axis=1)
# 计算刚体变换
mean_b = np.mean(point_cloud_b, axis=0)
mean_a = np.mean(transformed_point_cloud_a, axis=0)
centroid_distance = mean_a - np.dot(transform[:3, 3], transform[:3, :3].T) @ mean_b
rotation_matrix, translation = cv2.Rodrigues(np.dot(transform[:3, :3].T, transform[:3, :3] - np.eye(3)))[:2]
# 更新变换
transform[:3, :3] = rotation_matrix
transform[:3, 3] = translation + centroid_distance
# 检查是否达到精度要求
if np.linalg.norm(np.abs(transform[:3, :3] - np.eye(3))) < tolerance:
break
return transform
# 假设point_cloud_a和point_cloud_b是已经准备好的点云数据
transformed_point_cloud_a = icp(point_cloud_a, point_cloud_b)
# ...后续处理,如可视化、保存结果等
```
注意,这个示例非常基础,实际应用中可能还需要考虑更多的细节,如数据预处理、异常处理、以及更复杂的优化步骤。此外,`cv2.Rodrigues`用于从旋转变换矩阵转为欧拉角或其他形式。
相关推荐
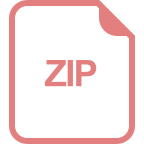
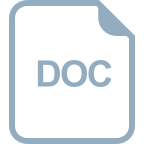
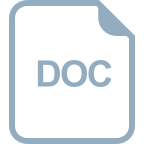
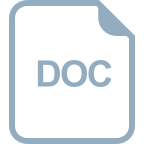
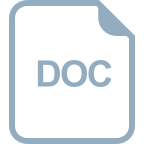
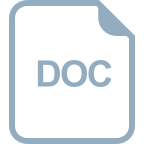









