用c语言写串口接收数据并分成两组代码
时间: 2024-09-27 13:08:54 浏览: 37
在C语言中,通过串口通信接收数据通常涉及到硬件相关的头文件(如`<stdio.h>`、`<fcntl.h>`、`<termios.h>` 和 `<unistd.h>` 等),以及一些库函数。下面是一个简单的示例,展示了如何打开串口,设置波特率,然后读取数据并分隔成两组:
```c
#include <stdio.h>
#include <fcntl.h>
#include <termios.h>
#include <unistd.h>
#define SERIAL_PORT "/dev/ttyUSB0" // 这里替换为实际的串口路径
#define BAUD_RATE B9600
int main() {
int fd;
struct termios options;
// 打开串口
if ((fd = open(SERIAL_PORT, O_RDWR | O_NOCTTY)) == -1) {
perror("Failed to open serial port");
return -1;
}
// 设置端口属性
tcgetattr(fd, &options);
cfsetispeed(&options, BAUD_RATE);
cfsetospeed(&options, BAUD_RATE);
options.c_cflag &= ~CSIZE; // 清除位宽标志
options.c_cflag |= CS8; // 设置为8位数据
options.c_iflag &= ~(IXON | IXOFF); // 关闭硬件流控
options.c_lflag &= ~(ICANON | ECHO); // 关闭终端处理和回显
options.c_oflag &= ~OPOST; // 关闭发送缓冲区
tcflush(fd, TCIFLUSH); // 清空输入缓冲区
char buffer[256];
ssize_t bytes_received;
while (true) {
bytes_received = read(fd, buffer, sizeof(buffer));
if (bytes_received <= 0) {
perror("Error reading from serial port");
break;
}
// 分割数据到两组
char group1[128], group2[128];
size_t group1_size = min(bytes_received, sizeof(group1));
size_t group2_size = bytes_received - group1_size;
memcpy(group1, buffer, group1_size);
memcpy(&buffer[group1_size], group2, group2_size);
// 处理接收到的数据...
printf("Group 1: %s\n", group1);
printf("Group 2: %s\n", group2);
}
close(fd);
return 0;
}
```
请注意,这只是一个基本示例,实际应用中可能需要考虑更复杂的错误处理和数据解析。此外,上述代码依赖于Linux环境,对于其他平台可能需要相应调整。
阅读全文
相关推荐
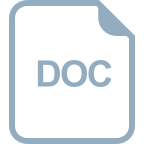
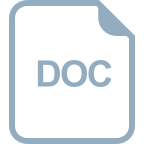
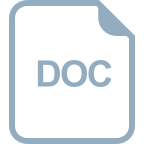
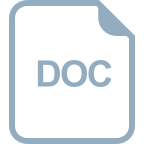

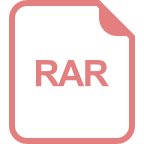
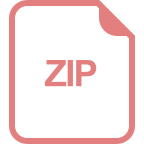
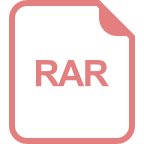
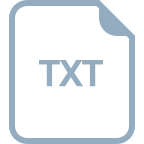
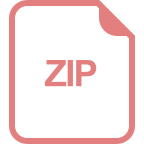
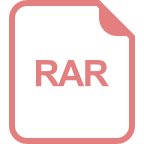
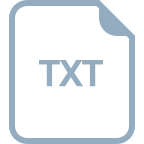
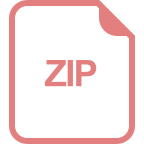
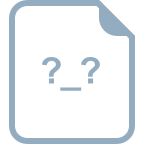
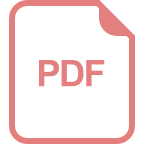
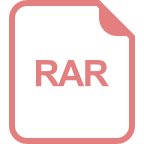
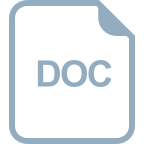
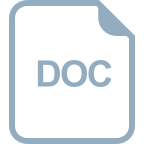