c语言链表双循环求静态串所含不同字符的种类数和每种字符个数具体代码
时间: 2024-10-29 21:25:17 浏览: 18
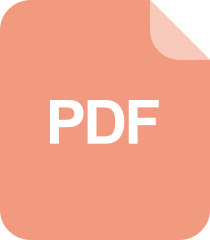
C语言难点及分析
在 C 语言中,我们可以使用链表数据结构来存储字符串,并通过两个指针分别遍历链表,一个用于正向遍历,另一个用于反向遍历,以便找出所有不同的字符及其计数。这里是一个简单的示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
// 定义链表节点结构
typedef struct Node {
char data;
struct Node* next;
} Node;
// 创建新节点
Node* createNode(char data) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = data;
newNode->next = NULL;
return newNode;
}
// 添加元素到链表末尾
void append(Node** head, char data) {
if (*head == NULL) {
*head = createNode(data);
} else {
Node* current = *head;
while (current->next != NULL)
current = current->next;
current->next = createNode(data);
}
}
// 双向遍历计算字符种类和数量
void countChars(Node* start, Node* end, int* charCount, int* charTypes) {
int type = 0; // 当前字符类型
for (Node* curr = start; curr != end; curr = curr->next) {
if (curr->data != start->data) { // 避免重复计数第一个字符
++charCount[type];
if (type >= *charTypes) {
++(*charTypes); // 如果达到当前已记录类型的上限,增加类型数
}
}
if (curr == end || curr->data != curr->next->data) { // 判断是否到达链表头或下一个字符不同
type = curr->data; // 更新当前字符类型
}
}
}
int main() {
char string[100]; // 假设输入字符串在这里
fgets(string, sizeof(string), stdin); // 读取用户输入
Node* head = NULL;
Node* tail = NULL;
for (size_t i = 0; i < strlen(string); ++i) {
append(&head, string[i]);
if (tail != NULL)
tail->next = NULL; // 尾部节点不需要指向下一个
tail = head;
}
Node* start = head;
Node* end = head;
for (end = tail; end != head; end = end->next) { // 反向遍历找到头节点
start = start->next;
}
int charTypes = 1; // 初始化字符类型为1(包括第一个字符)
int charCount[charTypes];
countChars(start, end, charCount, &charTypes);
printf("不同字符的种类数: %d\n", charTypes);
for (int i = 0; i < charTypes; ++i) {
printf("字符 '%c' 出现了 %d 次。\n", 'a' + i, charCount[i]); // 假定字符集是小写字母 a-z
}
return 0;
}
```
这个程序首先创建一个链表来存储输入的字符串,然后从两端同时开始遍历,统计每个不同字符的出现次数。注意,这里的代码假设输入字符串只包含小写字母,并且类型数不超过 `26`。
阅读全文
相关推荐
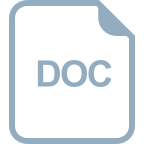
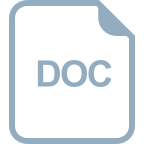
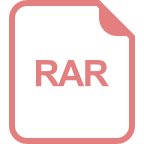
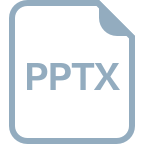
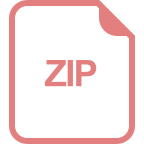
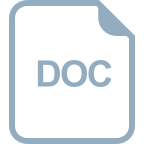
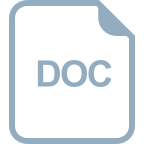
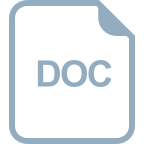
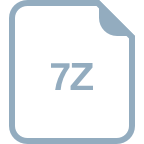
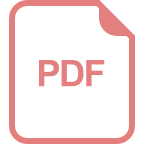
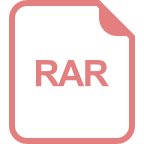
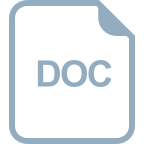
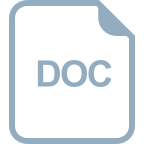
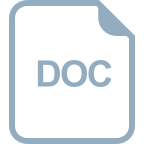
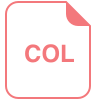
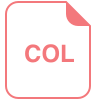
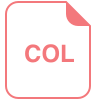
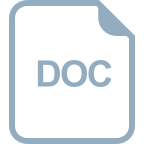