3.拓展题:表达式求值程序: 要求:键盘输入一个中缀表达式,判断表达式的括号是否匹配,如匹配,把中缀表达式转换为后缀表达式,并对后缀表达式求值,输出表达式的值。要求在devc++5.11下写出代码
时间: 2024-11-13 12:26:25 浏览: 4
在Dev-C++ 5.11下,我们可以使用栈的数据结构来完成这个任务,包括检查括号匹配、转换为后缀表达式(也称逆波兰表达式,RPN)以及计算其值。以下是一个基本的实现:
```cpp
#include <iostream>
#include <stack>
#include <string>
#include <sstream>
bool isOperator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/';
}
bool isValidParentheses(const string& exp) {
stack<char> parentheses;
for (size_t i = 0; i < exp.length(); ++i) {
if (exp[i] == '(') {
parentheses.push('(');
} else if (exp[i] == ')') {
if (parentheses.empty() || parentheses.top() != '(') {
return false;
}
parentheses.pop();
}
}
return parentheses.empty();
}
string infixToPostfix(const string& exp) {
stack<char> operators;
stringstream ss(exp);
string postfixExp;
char op;
while (ss >> op) {
if (isOperator(op)) {
while (!operators.empty() && precedence(op) <= precedence(operators.top())) {
postfixExp += operators.top();
operators.pop();
}
operators.push(op);
} else {
postfixExp += op;
}
}
while (!operators.empty()) {
postfixExp += operators.top();
operators.pop();
}
return postfixExp;
}
double evaluatePostfix(string postfixExp) {
stack<double> values;
for (char c : postfixExp) {
if (isdigit(c)) {
double value = 0;
while (isdigit(postfixExp.back())) {
value *= 10;
value += c - '0';
postfixExp.pop_back();
}
values.push(value);
} else {
double operand2 = values.top();
values.pop();
double operand1 = values.top();
values.pop();
switch (c) {
case '+':
values.push(operand1 + operand2);
break;
case '-':
values.push(operand1 - operand2);
break;
case '*':
values.push(operand1 * operand2);
break;
case '/':
if (operand2 != 0)
values.push(operand1 / operand2);
else
throw runtime_error("Division by zero not allowed");
break;
}
}
}
return values.top();
}
int main() {
string expression;
cout << "Enter an expression with balanced parentheses: ";
getline(cin, expression);
if (isValidParentheses(expression)) {
string postfixExpression = infixToPostfix(expression);
try {
double result = evaluatePostfix(postfixExpression);
cout << "The value of the expression is: " << result << endl;
} catch (const exception& e) {
cerr << "Error: " << e.what() << endl;
}
} else {
cerr << "Invalid expression, please ensure proper parentheses matching." << endl;
}
return 0;
}
```
此代码首先读取用户输入的中缀表达式,然后检查括号是否匹配。如果匹配,程序继续将中缀表达式转换为后缀表达式,然后计算其值。
注意:这个版本的代码只支持加减乘除运算,并假设输入的表达式不含除零错误。如果需要更复杂的支持,比如优先级更高的运算符或其他特殊函数,可以扩展`precedence()`和`evaluatePostfix()`函数。
阅读全文
相关推荐
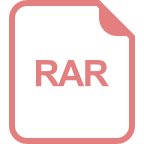
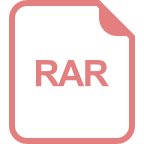
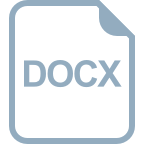
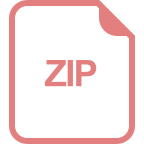
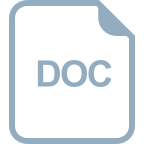












