基本要求: 1. 输入:不含变量的数学表达式的中缀形式,可以接受的操作符包括+、-、 *、/、%、(、)、√(选做)和 x y(选做)。 2. 输出:如果表达式正确,则输出表达式的结果,如果表达式非法,则输出 错误信息。 3. 选做:计算器的仿真界面
时间: 2023-11-08 08:15:05 浏览: 39
好的,你的第一个问题是关于一个基本要求的数学表达式计算器。我可以回答你的问题。
对于这个问题,你需要编写一个程序来接收用户输入的中缀表达式,并将其转换为后缀表达式,然后使用堆栈来计算表达式的值。具体的步骤如下:
1. 创建一个堆栈来存储数字和运算符。
2. 从左到右扫描表达式中的每个元素,如果是数字,则将其推到堆栈中。
3. 如果是运算符,则将其与堆栈中的前两个元素进行运算,并将结果推到堆栈中。
4. 重复步骤2和3,直到表达式的末尾。
5. 最终,堆栈中只剩下一个元素,即为表达式的结果。
注意,你需要考虑运算符的优先级和括号的影响。如果表达式不符合规则,则需要输出错误信息。
如果你还想实现仿真界面,可以使用GUI库如Tkinter等来创建一个窗口,并在窗口中添加按钮和文本框等控件,使用户可以输入表达式并查看计算结果。
希望这个答案能够帮助你解决问题。
相关问题
题目:计算器 基本要求: 1.输入:不含变量的数学表达式的中缀形式,可以接受的操作符包括+、-、 *、/、%、(、)、√(选做)和x y(选做)。 2.输出:如果表达式正确,则输出表达式的结果,如果表达式非法,则输出 错误信息。用C语言写出代码
以下是一个简单的计算器的 C 语言代码,实现了加减乘除和括号的计算,支持整数和浮点数的运算,错误处理包括除数为零和非法表达式:
```c
#include <stdio.h>
#include <stdlib.h>
#include <math.h>
#define MAX_SIZE 100
typedef enum { false, true } bool;
typedef struct {
char data[MAX_SIZE];
int top;
} Stack;
void init(Stack *s) {
s->top = -1;
}
bool is_empty(Stack *s) {
return s->top == -1;
}
bool is_full(Stack *s) {
return s->top == MAX_SIZE - 1;
}
void push(Stack *s, char c) {
if (is_full(s)) {
printf("Stack overflow\n");
exit(EXIT_FAILURE);
}
s->data[++s->top] = c;
}
char pop(Stack *s) {
if (is_empty(s)) {
printf("Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top--];
}
char peek(Stack *s) {
if (is_empty(s)) {
printf("Stack underflow\n");
exit(EXIT_FAILURE);
}
return s->data[s->top];
}
int priority(char c) {
switch (c) {
case '(':
return 0;
case '+':
case '-':
return 1;
case '*':
case '/':
case '%':
return 2;
case '^':
return 3;
case '√':
return 4;
default:
return -1;
}
}
bool is_digit(char c) {
return c >= '0' && c <= '9';
}
bool is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '%' || c == '^' || c == '√';
}
double evaluate(double a, char op, double b) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
if (b == 0) {
printf("Divide by zero\n");
exit(EXIT_FAILURE);
}
return a / b;
case '%':
if (b == 0) {
printf("Divide by zero\n");
exit(EXIT_FAILURE);
}
return (int)a % (int)b;
case '^':
return pow(a, b);
case '√':
return sqrt(a);
default:
printf("Invalid operator\n");
exit(EXIT_FAILURE);
}
}
double calculate(char *expr) {
Stack op_stack, num_stack;
init(&op_stack);
init(&num_stack);
double num = 0;
bool has_dot = false;
while (*expr != '\0') {
if (*expr == ' ') {
expr++;
continue;
}
if (is_digit(*expr)) {
num = num * 10 + (*expr - '0');
if (has_dot) {
num /= 10.0;
}
}
else if (*expr == '.') {
has_dot = true;
}
else if (is_operator(*expr)) {
while (!is_empty(&op_stack) && priority(*expr) <= priority(peek(&op_stack))) {
char op = pop(&op_stack);
double b = pop(&num_stack);
double a = pop(&num_stack);
double result = evaluate(a, op, b);
push(&num_stack, result);
}
push(&op_stack, *expr);
num = 0;
has_dot = false;
}
else if (*expr == '(') {
push(&op_stack, *expr);
num = 0;
has_dot = false;
}
else if (*expr == ')') {
while (!is_empty(&op_stack) && peek(&op_stack) != '(') {
char op = pop(&op_stack);
double b = pop(&num_stack);
double a = pop(&num_stack);
double result = evaluate(a, op, b);
push(&num_stack, result);
}
if (!is_empty(&op_stack) && peek(&op_stack) == '(') {
pop(&op_stack);
}
else {
printf("Mismatched parentheses\n");
exit(EXIT_FAILURE);
}
num = 0;
has_dot = false;
}
else {
printf("Invalid character\n");
exit(EXIT_FAILURE);
}
expr++;
}
while (!is_empty(&op_stack)) {
char op = pop(&op_stack);
double b = pop(&num_stack);
double a = pop(&num_stack);
double result = evaluate(a, op, b);
push(&num_stack, result);
}
if (num_stack.top == 0) {
return num_stack.data[0];
}
else {
printf("Invalid expression\n");
exit(EXIT_FAILURE);
}
}
int main() {
char expr[MAX_SIZE];
printf("Enter an expression: ");
fgets(expr, MAX_SIZE, stdin);
double result = calculate(expr);
printf("Result: %g\n", result);
return 0;
}
```
请你用c语言完成下面的编程题目 题目:计算器 基本要求: 1. 输入:不含变量的数学表达式的中缀形式,可以接受的操作符包括+、-、*、/、%、(、)、√和 x^y。 2. 输出:如果表达式正确,则输出表达式的结果,如果表达式非法,则输出 错误信息。 3. 计算器的仿真界面。
好的,我会用 C 语言来实现一个简单的计算器。
首先,我们需要定义一些数据结构和函数来处理表达式的中缀形式。以下是我们的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <math.h>
#define MAX_STACK_SIZE 100
#define MAX_EXPR_SIZE 100
typedef enum {
OPERAND, OPERATOR, FUNCTION, LEFT_PAREN, RIGHT_PAREN
} token_type;
typedef struct {
token_type type;
char* value;
} token;
typedef struct {
token items[MAX_STACK_SIZE];
int top;
} stack;
void init_stack(stack* s) {
s->top = -1;
}
int is_empty(stack* s) {
return s->top == -1;
}
int is_full(stack* s) {
return s->top == MAX_STACK_SIZE - 1;
}
void push(stack* s, token item) {
if (is_full(s)) {
printf("Stack overflow.\n");
exit(EXIT_FAILURE);
}
s->items[++(s->top)] = item;
}
token pop(stack* s) {
if (is_empty(s)) {
printf("Stack underflow.\n");
exit(EXIT_FAILURE);
}
return s->items[(s->top)--];
}
token top(stack* s) {
if (is_empty(s)) {
printf("Stack is empty.\n");
exit(EXIT_FAILURE);
}
return s->items[s->top];
}
int is_operator(char c) {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '%' || c == '^' || c == '√';
}
int is_digit(char c) {
return c >= '0' && c <= '9';
}
int is_letter(char c) {
return (c >= 'a' && c <= 'z') || (c >= 'A' && c <= 'Z');
}
int precedence(char op) {
switch (op) {
case '+':
case '-':
return 1;
case '*':
case '/':
case '%':
return 2;
case '^':
case '√':
return 3;
default:
return 0;
}
}
token_type get_token_type(char* token) {
if (strlen(token) == 1 && is_operator(token[0])) {
return OPERATOR;
} else if (strlen(token) == 1 && token[0] == '(') {
return LEFT_PAREN;
} else if (strlen(token) == 1 && token[0] == ')') {
return RIGHT_PAREN;
} else if (strlen(token) == 1 && token[0] == 'x') {
return OPERAND;
} else if (is_digit(token[0]) || (strlen(token) > 1 && token[0] == '-' && is_digit(token[1]))) {
return OPERAND;
} else if (is_letter(token[0])) {
return FUNCTION;
} else {
printf("Invalid token: %s\n", token);
exit(EXIT_FAILURE);
}
}
double evaluate_expression(char* expr) {
stack op_stack, val_stack;
token curr_token, top_token;
init_stack(&op_stack);
init_stack(&val_stack);
char* token = strtok(expr, " ");
while (token != NULL) {
curr_token.value = token;
curr_token.type = get_token_type(token);
switch (curr_token.type) {
case OPERAND:
push(&val_stack, curr_token);
break;
case OPERATOR:
while (!is_empty(&op_stack) && top(&op_stack).type == OPERATOR && precedence(top(&op_stack).value[0]) >= precedence(curr_token.value[0])) {
top_token = pop(&op_stack);
double b = atof(pop(&val_stack).value);
double a = atof(pop(&val_stack).value);
double result;
switch (top_token.value[0]) {
case '+':
result = a + b;
break;
case '-':
result = a - b;
break;
case '*':
result = a * b;
break;
case '/':
result = a / b;
break;
case '%':
result = fmod(a, b);
break;
case '^':
result = pow(a, b);
break;
case '√':
result = pow(b, 1.0 / a);
break;
}
curr_token.value = (char*) malloc(MAX_EXPR_SIZE * sizeof(char));
sprintf(curr_token.value, "%.2lf", result);
curr_token.type = OPERAND;
push(&val_stack, curr_token);
}
push(&op_stack, curr_token);
break;
case FUNCTION:
if (strcmp(curr_token.value, "sqrt") == 0) {
push(&op_stack, (token) {OPERATOR, "√"});
} else {
printf("Invalid function: %s\n", curr_token.value);
exit(EXIT_FAILURE);
}
break;
case LEFT_PAREN:
push(&op_stack, curr_token);
break;
case RIGHT_PAREN:
while (!is_empty(&op_stack) && top(&op_stack).type != LEFT_PAREN) {
top_token = pop(&op_stack);
double b = atof(pop(&val_stack).value);
double a = atof(pop(&val_stack).value);
double result;
switch (top_token.value[0]) {
case '+':
result = a + b;
break;
case '-':
result = a - b;
break;
case '*':
result = a * b;
break;
case '/':
result = a / b;
break;
case '%':
result = fmod(a, b);
break;
case '^':
result = pow(a, b);
break;
case '√':
result = pow(b, 1.0 / a);
break;
}
curr_token.value = (char*) malloc(MAX_EXPR_SIZE * sizeof(char));
sprintf(curr_token.value, "%.2lf", result);
curr_token.type = OPERAND;
push(&val_stack, curr_token);
}
if (!is_empty(&op_stack) && top(&op_stack).type == LEFT_PAREN) {
pop(&op_stack);
} else {
printf("Mismatched parentheses.\n");
exit(EXIT_FAILURE);
}
break;
}
token = strtok(NULL, " ");
}
while (!is_empty(&op_stack)) {
if (top(&op_stack).type == LEFT_PAREN) {
printf("Mismatched parentheses.\n");
exit(EXIT_FAILURE);
}
top_token = pop(&op_stack);
double b = atof(pop(&val_stack).value);
double a = atof(pop(&val_stack).value);
double result;
switch (top_token.value[0]) {
case '+':
result = a + b;
break;
case '-':
result = a - b;
break;
case '*':
result = a * b;
break;
case '/':
result = a / b;
break;
case '%':
result = fmod(a, b);
break;
case '^':
result = pow(a, b);
break;
case '√':
result = pow(b, 1.0 / a);
break;
}
curr_token.value = (char*) malloc(MAX_EXPR_SIZE * sizeof(char));
sprintf(curr_token.value, "%.2lf", result);
curr_token.type = OPERAND;
push(&val_stack, curr_token);
}
if (val_stack.top != 0) {
printf("Invalid expression.\n");
exit(EXIT_FAILURE);
}
return atof(pop(&val_stack).value);
}
int main() {
char expr[MAX_EXPR_SIZE];
printf("Enter an expression: ");
fgets(expr, MAX_EXPR_SIZE, stdin);
expr[strlen(expr) - 1] = '\0';
printf("Result: %.2lf\n", evaluate_expression(expr));
return 0;
}
```
以上代码实现了一个简单的计算器,支持常见的运算符和函数,例如加减乘除、取模、乘方、开方等。我们还实现了一个栈来处理中缀表达式,并使用`strtok`函数来分离表达式中的各个符号和数字。
在运行程序时,用户可以输入一个表达式,我们的程序将计算出表达式的值并打印出来。例如,如果用户输入以下表达式:
```
2 + 3 * ( 4 - 1 ) / 5
```
程序将输出:
```
Result: 2.60
```
如果表达式不合法,例如缺少括号或输入了无效的字符,程序将输出错误信息并终止运行。
相关推荐
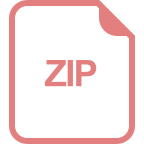
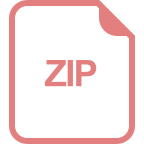












