python爬虫爬取b站弹幕评论
时间: 2024-12-24 20:04:06 浏览: 7
要使用Python爬虫爬取B站(哔哩哔哩)的弹幕和评论,可以按照以下步骤进行:
### 1. 准备工作
首先,确保你已经安装了必要的Python库:
```bash
pip install requests
pip install beautifulsoup4
pip install selenium
```
### 2. 获取弹幕和评论的API
B站的弹幕和评论数据通常通过API接口提供。我们需要找到这些接口的URL。
#### 获取弹幕
弹幕数据通常可以通过以下URL获取:
```
https://comment.bilibili.com/{cid}.xml
```
其中,`{cid}`是视频的唯一标识符。你可以通过视频页面的HTML代码中找到这个`cid`。
#### 获取评论
评论数据可以通过以下URL获取:
```
https://api.bilibili.com/x/v1/dm/list.so?oid={cid}
```
或者通过其他API接口,具体取决于你要获取的评论类型。
### 3. 编写爬虫代码
以下是一个简单的示例代码,展示如何使用`requests`和`BeautifulSoup`库来爬取弹幕和评论:
```python
import requests
from bs4 import BeautifulSoup
def get_cid(url):
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
cid = soup.find('meta', property='og:url')['content'].split('/')[-1]
return cid
def get_danmaku(cid):
url = f'https://comment.bilibili.com/{cid}.xml'
response = requests.get(url)
return response.text
def get_comments(cid):
url = f'https://api.bilibili.com/x/v1/dm/list.so?oid={cid}'
response = requests.get(url)
return response.text
if __name__ == "__main__":
video_url = 'https://www.bilibili.com/video/BV1xJ411X7Y1' # 替换为你要爬取的B站视频URL
cid = get_cid(video_url)
danmaku = get_danmaku(cid)
comments = get_comments(cid)
print('弹幕数据:')
print(danmaku)
print('评论数据:')
print(comments)
```
### 4. 处理数据
根据需要,你可以使用`BeautifulSoup`或其他解析库来处理获取到的XML或JSON数据,提取出有用的信息。
### 5. 注意事项
1. **合法性**:爬虫行为可能违反B站的使用条款,请确保你的行为合法且不侵犯他人权益。
2. **反爬机制**:B站可能有反爬机制,如IP限制、验证码等。可以考虑使用代理、延时请求等方法来规避。
3. **数据存储**:根据需要,将爬取到的数据存储到文件或数据库中。
阅读全文
相关推荐
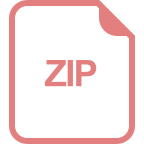
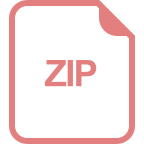
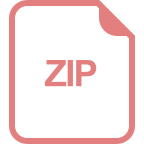



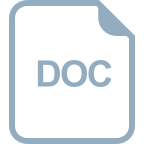




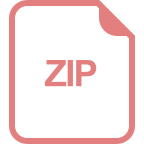






