改成用c语言或c++实现
时间: 2024-12-24 22:41:06 浏览: 6
在 C 语言或 C++ 中实现上述过程,你需要依赖于库函数如 FFTW 或者 OpenCV 提供的接口。以下是基于 C 语言的一个简单示例,使用 FFTW 库进行低通滤波:
```c
#include <fftw3.h>
#include <stdio.h>
#include <stdlib.h>
// 函数声明
void apply_low_pass_filter(int img[height][width], int out[height][width], int filter_width, double cut_off_freq);
void fft_transform_and_filter(int input[], int output[], int size);
int main() {
// 读取和预处理图像
const int width = 640;
const int height = 480;
unsigned char img[height][width];
...
// 转换为灰度图像
for (int y = 0; y < height; ++y) {
for (int x = 0; x < width; ++x) {
img[y][x] = img_gray[y][x];
}
}
// 初始化滤波器宽度和截止频率
int filter_width = 5;
double cut_off_freq;
// 应用低通滤波
int filtered_img[height][width];
apply_low_pass_filter(img, filtered_img, filter_width, cut_off_freq);
// 输出处理后的图像
...
return 0;
}
// 使用FFTW进行离散傅立叶变换
void fft_transform_and_filter(int input[], int output[], int size) {
fftw_complex* in = (fftw_complex*) input;
fftw_complex* out = (fftw_complex*) output;
fftw_plan plan = fftw_plan_dft_r2c_1d(size, in, out, FFTW_ESTIMATE);
fftw_execute(plan);
fftw_destroy_plan(plan);
}
// 实现低通滤波
void apply_low_pass_filter(int img[height][width], int out[height][width], int filter_width, double cut_off_freq) {
int N = height * width;
fftw_complex* dft_img = (fftw_complex*) malloc(sizeof(fftw_complex) * N);
fftw_complex* dft_filtered = (fftw_complex*) malloc(sizeof(fftw_complex) * N);
// 将输入图像转换到复数形式
for (int i = 0; i < N; i++) {
dft_img[i][0] = (double) img[i];
dft_img[i][1] = 0.0;
}
// 执行DFT
fft_transform_and_filter(dft_img, dft_filtered, N);
// 简单的高斯滤波(这里仅示例,实际应使用更复杂的方法)
for (int k = 0; k < N / 2 + 1; ++k) {
if (k < filter_width / 2 || (N - k) < filter_width / 2) {
double freq = (double) k * (2 * M_PI) / N;
if (freq <= cut_off_freq) {
dft_filtered[k][0] *= exp(-((freq - cut_off_freq) * (freq - cut_off_freq)) / (2 * sigma * sigma));
dft_filtered[k][1] = 0.0;
} else {
dft_filtered[k][0] = 0.0;
}
} else {
dft_filtered[k][0] = 0.0;
}
}
// 取实部并将结果放回原数组
for (int i = 0; i < N; i++) {
out[i] = (double)(dft_filtered[i][0]);
}
free(dft_img);
free(dft_filtered);
}
```
别忘了在实际项目中链接相应的 FFTW 库文件和适当初始化 FFTW。
阅读全文
相关推荐
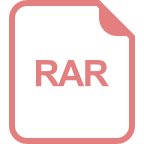
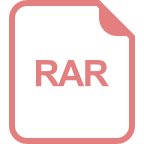


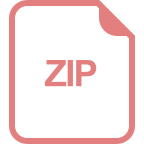
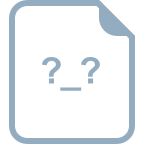

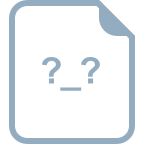
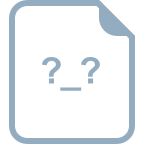
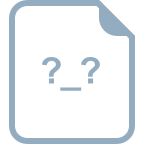






