c++ 模拟退火求函数最小值
时间: 2023-08-03 10:06:07 浏览: 127
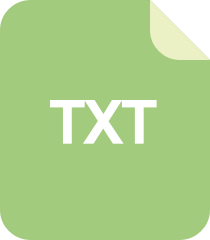
模拟退火算法(Simulated Annealing Algorithm)是一种全局优化算法,其灵感来源于固体退火过程
你可以使用C++来编写模拟退火算法来求解函数的最小值。下面是一个简单的示例代码:
```cpp
#include <iostream>
#include <cmath>
#include <cstdlib>
#include <ctime>
double objectiveFunction(double x) {
// 这里是你要求解的函数,可以根据实际情况修改
return sin(x);
}
double simulatedAnnealing(double initialTemp, double finalTemp, double coolingRate, double xLowerBound, double xUpperBound) {
// 随机生成初始解
double currentSolution = xLowerBound + static_cast<double>(rand()) / RAND_MAX * (xUpperBound - xLowerBound);
double bestSolution = currentSolution;
// 计算初始解的目标函数值
double currentEnergy = objectiveFunction(currentSolution);
double bestEnergy = currentEnergy;
// 开始模拟退火过程
double temperature = initialTemp;
while (temperature > finalTemp) {
// 生成邻域解
double newSolution = xLowerBound + static_cast<double>(rand()) / RAND_MAX * (xUpperBound - xLowerBound);
double newEnergy = objectiveFunction(newSolution);
// 计算能量差
double energyDiff = newEnergy - currentEnergy;
// 如果新解更优,则接受新解
if (energyDiff < 0) {
currentSolution = newSolution;
currentEnergy = newEnergy;
}
// 否则以一定概率接受新解
else if (exp(-energyDiff / temperature) > static_cast<double>(rand()) / RAND_MAX) {
currentSolution = newSolution;
currentEnergy = newEnergy;
}
// 更新最优解
if (currentEnergy < bestEnergy) {
bestSolution = currentSolution;
bestEnergy = currentEnergy;
}
// 降低温度
temperature *= coolingRate;
}
return bestSolution;
}
int main() {
// 设置随机数种子
srand(static_cast<unsigned int>(time(nullptr)));
// 调用模拟退火函数
double initialTemp = 100.0;
double finalTemp = 0.1;
double coolingRate = 0.95;
double xLowerBound = -10.0;
double xUpperBound = 10.0;
double result = simulatedAnnealing(initialTemp, finalTemp, coolingRate, xLowerBound, xUpperBound);
std::cout << "最小值在 x = " << result << " 处,函数值为 " << objectiveFunction(result) << std::endl;
return 0;
}
```
在上面的代码中,`objectiveFunction` 函数代表了你要求解的目标函数。`simulatedAnnealing` 函数实现了模拟退火算法。通过调整参数,可以对算法的性能进行调优。
在 `main` 函数中,你可以设置初始温度、最终温度、冷却速率以及变量的范围。最终,程序会输出找到的最小值所对应的变量值和函数值。
请注意,这只是一个简单的示例,你可以根据实际需求进行修改和优化。
阅读全文
相关推荐
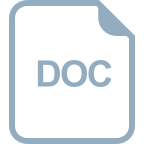
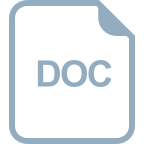
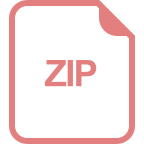
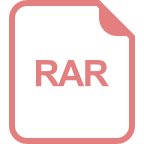
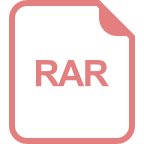
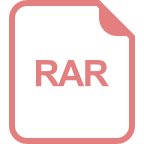
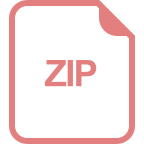
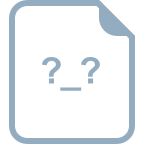
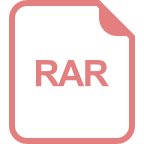
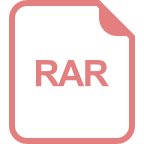
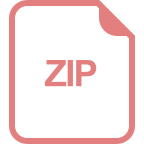
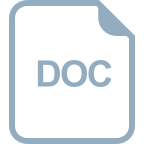
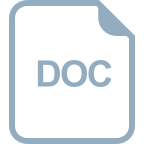
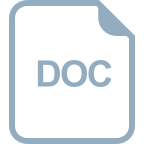
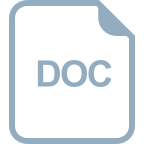
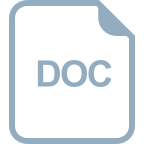
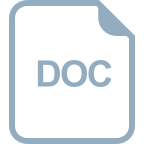
