基于CBC模式进行SM4的加解密操作,python代码进行注释说明
时间: 2024-05-14 18:19:54 浏览: 13
以下是基于CBC模式进行SM4的加解密操作的Python代码,代码中有详细的注释说明:
```python
from Crypto.Cipher import SM4
from Crypto.Util.Padding import pad, unpad
import binascii
# 定义加密函数
def encrypt_CBC(key, iv, plaintext):
# 将key转换为二进制格式
key = binascii.unhexlify(key)
# 将iv转换为二进制格式
iv = binascii.unhexlify(iv)
# 创建SM4加密器
cipher = SM4.new(key, SM4.MODE_CBC, iv)
# 对明文进行填充
plaintext = pad(plaintext.encode(), SM4.block_size)
# 加密
ciphertext = cipher.encrypt(plaintext)
# 将加密结果转换为16进制字符串
return binascii.hexlify(ciphertext).decode()
# 定义解密函数
def decrypt_CBC(key, iv, ciphertext):
# 将key转换为二进制格式
key = binascii.unhexlify(key)
# 将iv转换为二进制格式
iv = binascii.unhexlify(iv)
# 创建SM4解密器
cipher = SM4.new(key, SM4.MODE_CBC, iv)
# 将密文转换为二进制格式
ciphertext = binascii.unhexlify(ciphertext)
# 解密
plaintext = cipher.decrypt(ciphertext)
# 对解密结果进行去填充
return unpad(plaintext, SM4.block_size).decode()
# 测试
key = '0123456789abcdeffedcba9876543210'
iv = '0123456789abcdef0123456789abcdef'
plaintext = 'hello, world!'
print('plaintext:', plaintext)
# 加密
ciphertext = encrypt_CBC(key, iv, plaintext)
print('ciphertext:', ciphertext)
# 解密
plaintext = decrypt_CBC(key, iv, ciphertext)
print('plaintext:', plaintext)
```
其中,`Crypto.Cipher`模块提供了SM4加解密器的实现,`Crypto.Util.Padding`模块提供了填充和去填充的函数。在加密和解密函数中,需要将密钥、初始向量和明文/密文转换为二进制格式,使用SM4加解密器进行加解密操作,最后再将结果转换为16进制字符串或明文。在测试代码中,先定义了密钥、初始向量和明文,然后调用加密函数进行加密操作,再调用解密函数进行解密操作,最后输出解密后的明文。
相关推荐
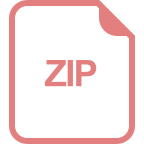
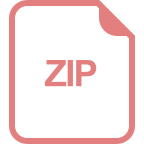














