C++授权文件的实现代码
时间: 2023-06-12 12:08:23 浏览: 178
C++授权文件的实现可以通过以下步骤实现:
1. 生成授权文件:可以使用加密算法(如AES)对授权信息进行加密,并将加密后的信息写入文件中。
2. 验证授权文件:将授权文件中的信息解密,与程序中的授权信息进行比较,判断授权是否有效。
下面是一个简单的示例代码:
```c++
#include <iostream>
#include <fstream>
#include <string>
#include <openssl/aes.h>
// 加密密钥
const unsigned char key[] = {0x01, 0x23, 0x45, 0x67, 0x89, 0xab, 0xcd, 0xef,
0xfe, 0xdc, 0xba, 0x98, 0x76, 0x54, 0x32, 0x10};
// 授权信息
const std::string license = "20210930";
// 加密函数
void encrypt(const unsigned char* in, unsigned char* out, const unsigned char* key)
{
AES_KEY aes_key;
AES_set_encrypt_key(key, 128, &aes_key);
AES_encrypt(in, out, &aes_key);
}
// 解密函数
void decrypt(const unsigned char* in, unsigned char* out, const unsigned char* key)
{
AES_KEY aes_key;
AES_set_decrypt_key(key, 128, &aes_key);
AES_decrypt(in, out, &aes_key);
}
// 生成授权文件
void generate_license_file(const std::string& file_path)
{
unsigned char encrypted_license[16];
encrypt((const unsigned char*)license.c_str(), encrypted_license, key);
std::ofstream outfile(file_path, std::ios::out | std::ios::binary);
if (outfile)
{
outfile.write((const char*)encrypted_license, sizeof(encrypted_license));
outfile.close();
std::cout << "License file generated successfully!" << std::endl;
}
else
{
std::cerr << "Failed to generate license file!" << std::endl;
}
}
// 验证授权文件
bool verify_license_file(const std::string& file_path)
{
std::ifstream infile(file_path, std::ios::in | std::ios::binary);
if (infile)
{
unsigned char encrypted_license[16];
infile.read((char*)encrypted_license, sizeof(encrypted_license));
infile.close();
unsigned char decrypted_license[16];
decrypt(encrypted_license, decrypted_license, key);
std::string license_str((const char*)decrypted_license, sizeof(decrypted_license));
return (license_str == license);
}
else
{
std::cerr << "Failed to open license file!" << std::endl;
return false;
}
}
int main()
{
generate_license_file("license.dat");
bool is_valid = verify_license_file("license.dat");
std::cout << "License is " << (is_valid ? "valid" : "invalid") << std::endl;
return 0;
}
```
在以上示例代码中,使用了AES算法进行加密和解密。首先在程序中指定了加密密钥和授权信息,然后实现了加密和解密函数。在生成授权文件时,将授权信息进行加密,写入文件;在验证授权文件时,从文件中读取加密的授权信息,进行解密,并与程序中的授权信息进行比较,判断授权是否有效。
阅读全文
相关推荐
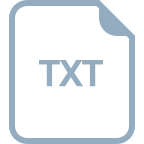
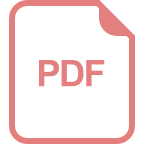
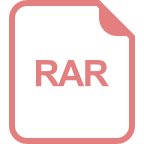
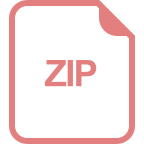
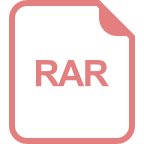
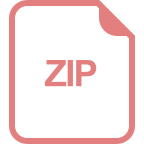
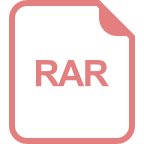
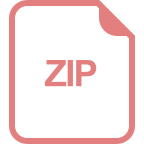
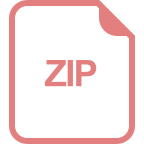
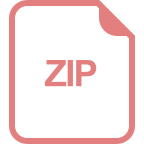
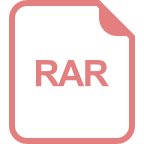
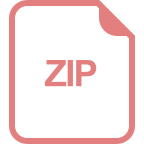
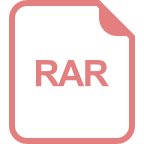
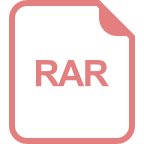
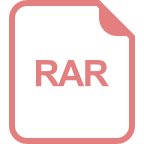
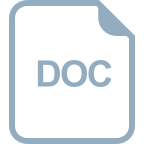
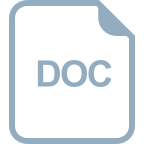