getList().then((res:UTSJSONObject)=>{ console.log('*********************',res?.data) })报错:error: Unresolved reference: data
时间: 2024-06-17 08:06:08 浏览: 96
这个报错可能是因为在 `res` 中找不到 `data` 属性。可能原因如下:
1. `res` 中确实没有 `data` 属性。
2. `data` 属性是存在的,但是没有使用正确的语法访问。
你可以检查一下返回的 `res` 对象是否有 `data` 属性。如果有,那么你需要检查一下访问 `data` 属性的语法是否正确。
此外,也可以检查一下代码中是否正确导入了相关的依赖库或模块。
相关问题
handleDelete(row){ this.$confirm('是否删除当前用户信息?', '提示', { confirmButtonText: '确定', cancelButtonText: '取消', type: 'warning' }).then(() => { myAxios({ url:'/deleteUsergoods', params:{ user:row.user } }).then(res =>{ console.log(res); this.getList(); }).catch(err =>{ console.log(err); }) }).catch(() => {}); }优化这段代码
这段代码可以进行如下优化:
1. 将确认框的文本内容和类型定义为常量,避免多处使用时重复定义。
2. 将网络请求的操作封装为一个独立的函数,避免代码重复。
3. 将网络请求成功后的操作封装为一个独立的函数,方便代码重用。
4. 使用 async/await 代替 then/catch,使代码更加简洁易懂。
以下是优化后的代码:
```
const confirmText = '是否删除当前用户信息?';
const confirmType = 'warning';
async function deleteUsergoods(user) {
try {
const res = await myAxios({
url: '/deleteUsergoods',
params: { user },
});
return res;
} catch (err) {
console.log(err);
throw err;
}
}
function handleDelete(row) {
this.$confirm(confirmText, '提示', {
confirmButtonText: '确定',
cancelButtonText: '取消',
type: confirmType,
}).then(async () => {
await deleteUsergoods(row.user);
console.log('删除成功');
this.getList();
}).catch(() => {});
}
```
在uniapp中,console.log(this.userList) 不起作用 。 function getList() { user.getUserList().then(res => { console.log('getUserList>>>>>>>>>>>') console.log(res) const userList = res.result.records; console.log(userList) console.log(this.userList) this.userList = userList; }).catch(error => { }) }
在 `getList` 函数中,`console.log(this.userList)` 不起作用的原因是 `this` 指向的是当前函数的执行上下文,而不是组件实例。因此,代码中的 `this.userList` 并不是组件实例中定义的 `userList` 数据,而是一个未定义的变量。
为了解决这个问题,你可以在 `getList` 函数中将组件实例赋值给一个变量,然后使用该变量来访问组件数据。以下是一个修改后的示例:
```
function getList() {
const vm = this // 将组件实例赋值给变量 vm
user.getUserList().then(res => {
console.log('getUserList>>>>>>>>>>>')
console.log(res)
const userList = res.result.records;
console.log(userList)
console.log(vm.userList) // 使用 vm 访问组件数据
vm.userList = userList; // 更新组件数据
}).catch(error => {
// 异常处理
})
}
```
在上面的示例中,我们将组件实例赋值给了变量 `vm`,然后使用 `vm.userList` 来访问组件数据。这样就能够正确地更新组件数据了。
相关推荐
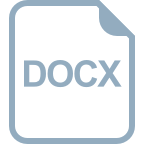
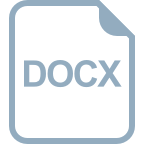
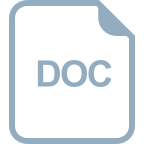
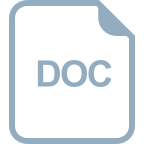
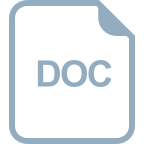
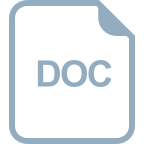
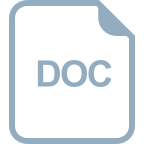







