python tensorflow dropout测试集
时间: 2023-08-28 14:06:03 浏览: 45
在使用 TensorFlow 训练模型时,可以使用 dropout 技术来防止过拟合。dropout 可以随机在神经网络中关闭一些神经元,从而减少神经元之间的依赖性,增加模型的泛化能力。在测试集上,我们需要关闭 dropout,因为我们希望模型使用所有的神经元来进行预测。
具体实现方法如下:
```python
import tensorflow as tf
# 定义模型
def model(x, training=True):
# 定义输入层
with tf.name_scope('input'):
input_layer = x
# 定义隐藏层
with tf.name_scope('hidden'):
hidden_layer = tf.layers.dense(inputs=input_layer, units=256, activation=tf.nn.relu)
# 使用 dropout
dropout_hidden_layer = tf.layers.dropout(hidden_layer, rate=0.5, training=training)
# 定义输出层
with tf.name_scope('output'):
output_layer = tf.layers.dense(inputs=dropout_hidden_layer, units=10)
return output_layer
# 定义输入和输出
x = tf.placeholder(tf.float32, [None, 784])
y = tf.placeholder(tf.float32, [None, 10])
# 构建模型
logits = model(x, training=True)
# 计算损失函数
with tf.name_scope('loss'):
cross_entropy = tf.reduce_mean(tf.nn.softmax_cross_entropy_with_logits_v2(labels=y, logits=logits))
# 定义优化器
with tf.name_scope('optimizer'):
train_step = tf.train.AdamOptimizer(learning_rate=0.001).minimize(cross_entropy)
# 计算准确率
with tf.name_scope('accuracy'):
correct_prediction = tf.equal(tf.argmax(logits, 1), tf.argmax(y, 1))
accuracy = tf.reduce_mean(tf.cast(correct_prediction, tf.float32))
# 创建会话
with tf.Session() as sess:
# 初始化变量
sess.run(tf.global_variables_initializer())
# 训练模型
for i in range(100):
batch_xs, batch_ys = mnist.train.next_batch(100)
sess.run(train_step, feed_dict={x: batch_xs, y: batch_ys})
# 在测试集上测试模型,关闭 dropout
acc = sess.run(accuracy, feed_dict={x: mnist.test.images, y: mnist.test.labels, training: False})
print("Test accuracy: %.4f" % acc)
```
在训练过程中,用 `training=True` 来启用 dropout。在测试过程中,用 `training=False` 来关闭 dropout。
相关推荐
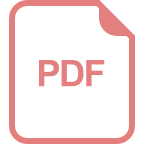













