gin 怎么对不同的表进行批量的增删改
时间: 2024-02-17 15:05:33 浏览: 91
在 Gin 框架中,通常需要使用 ORM(对象关系映射)库来操作数据库。常用的 ORM 库有 GORM、XORM、Beego ORM 等,这里以 GORM 为例来说明如何对不同的表进行批量的增删改。假设我们有三个表:User、Article、Comment。
首先,我们需要定义这三个表的结构体,例如:
```go
type User struct {
ID uint
Name string
Age int
}
type Article struct {
ID uint
Title string
Content string
}
type Comment struct {
ID uint
ArticleID uint
Content string
}
```
然后,我们可以使用 GORM 来对不同的表进行批量的增删改,例如:
```go
// 批量添加数据
users := []User{
{Name: "Alice", Age: 20},
{Name: "Bob", Age: 30},
{Name: "Charlie", Age: 40},
}
db.Create(&users)
articles := []Article{
{Title: "Gin tutorial", Content: "This is a Gin tutorial."},
{Title: "GORM tutorial", Content: "This is a GORM tutorial."},
{Title: "XORM tutorial", Content: "This is a XORM tutorial."},
}
db.Create(&articles)
comments := []Comment{
{ArticleID: 1, Content: "Nice tutorial!"},
{ArticleID: 2, Content: "Great tutorial!"},
{ArticleID: 3, Content: "Awesome tutorial!"},
}
db.Create(&comments)
// 批量修改数据
db.Model(&User{}).Where("age > ?", 30).Update("age", 50)
db.Model(&Article{}).Where("title LIKE ?", "%tutorial%").Update("content", "This is an updated tutorial.")
db.Model(&Comment{}).Where("id IN (?)", []int{1, 3}).Update("content", "This is an updated comment.")
// 批量删除数据
db.Where("age > ?", 50).Delete(&User{})
db.Where("title LIKE ?", "%tutorial%").Delete(&Article{})
db.Where("id IN (?)", []int{2, 3}).Delete(&Comment{})
```
在上面的代码中,我们分别对三个表进行了批量的增删改操作。例如,我们使用 `Create` 方法批量添加数据,使用 `Update` 方法批量修改数据,使用 `Delete` 方法批量删除数据。
需要注意的是,上面的代码假设我们已经成功连接到了数据库,并且已经通过 GORM 创建了相应的表。如果还没有创建相应的表,可以使用 GORM 的 `AutoMigrate` 方法来自动创建表。例如:
```go
// 自动创建表
db.AutoMigrate(&User{}, &Article{}, &Comment{})
```
阅读全文
相关推荐


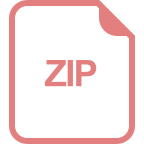
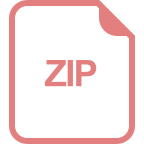
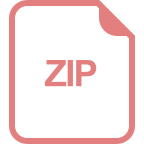
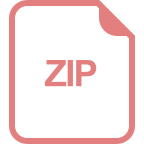
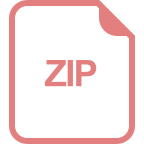
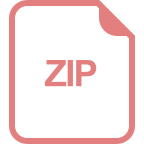
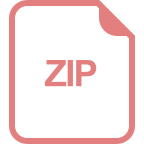
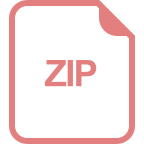
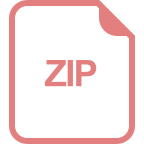
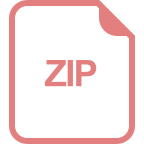
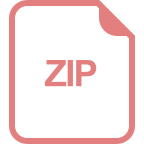
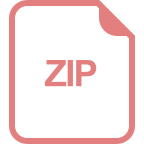
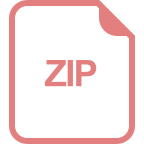
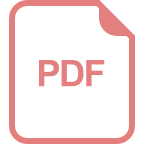