java使用多线程读取大文件,按顺序每次读50M的时候就用一个异步线程去加密这段数据,当所有数据读完后再按顺序合并
时间: 2023-12-18 11:02:05 浏览: 83
可以使用Java中的线程池来实现多线程读取大文件并异步加密。具体实现步骤如下:
1. 创建一个固定大小的线程池,例如使用`Executors.newFixedThreadPool(int n)`方法创建一个大小为n的线程池。
2. 定义一个`Runnable`接口的实现类`FileReader`,用于读取文件并进行加密处理。在该类中,可以使用`RandomAccessFile`类来读取大文件,每次读取50M的数据,然后异步加密处理。
3. 定义一个`Callable`接口的实现类`FileMerger`,用于将加密后的数据按顺序合并。在该类中,可以使用`RandomAccessFile`类将加密后的数据写入到新的文件中,并记录每个加密数据块的位置。
4. 在主线程中,先提交`FileReader`实例到线程池中执行,读取文件并异步加密。然后,等待所有加密任务执行完成后,再提交`FileMerger`实例到线程池中执行,将加密后的数据按顺序合并。
代码示例:
```
import java.io.File;
import java.io.RandomAccessFile;
import java.nio.ByteBuffer;
import java.nio.channels.FileChannel;
import java.util.ArrayList;
import java.util.List;
import java.util.concurrent.*;
public class FileEncryption {
private static final int BLOCK_SIZE = 50 * 1024 * 1024; // 每次读取50M数据
private static final int THREAD_POOL_SIZE = 4; // 线程池大小
private static final String INPUT_FILE_PATH = "/path/to/large/file"; // 待加密的大文件路径
private static final String OUTPUT_FILE_PATH = "/path/to/encrypted/file"; // 加密后的文件路径
private static ExecutorService executor = Executors.newFixedThreadPool(THREAD_POOL_SIZE); // 创建线程池
public static void main(String[] args) throws Exception {
List<Future<byte[]>> futures = new ArrayList<>(); // 存储加密任务的Future
try (RandomAccessFile inputFile = new RandomAccessFile(INPUT_FILE_PATH, "r");
FileChannel inputChannel = inputFile.getChannel()) {
long fileSize = inputFile.length();
long position = 0;
while (position < fileSize) {
long remaining = fileSize - position;
long blockSize = Math.min(remaining, BLOCK_SIZE);
ByteBuffer buffer = ByteBuffer.allocate((int) blockSize);
inputChannel.read(buffer, position);
position += blockSize;
buffer.flip();
Future<byte[]> future = executor.submit(new FileReader(buffer)); // 提交加密任务到线程池
futures.add(future);
}
}
List<byte[]> encryptedBlocks = new ArrayList<>();
for (Future<byte[]> future : futures) {
encryptedBlocks.add(future.get()); // 等待所有加密任务完成
}
executor.shutdown(); // 关闭线程池
try (RandomAccessFile outputFile = new RandomAccessFile(OUTPUT_FILE_PATH, "rw");
FileChannel outputChannel = outputFile.getChannel()) {
long position = 0;
for (byte[] block : encryptedBlocks) {
ByteBuffer buffer = ByteBuffer.wrap(block);
outputChannel.write(buffer, position); // 将加密后的数据按顺序写入到新文件中
position += block.length;
}
}
}
static class FileReader implements Callable<byte[]> {
private ByteBuffer buffer;
FileReader(ByteBuffer buffer) {
this.buffer = buffer;
}
@Override
public byte[] call() throws Exception {
// TODO: 进行加密处理
return buffer.array();
}
}
}
```
需要注意的是,由于使用了异步加密和多线程读取,加密后的数据块可能不是按原始文件的顺序排列的,因此需要在合并加密数据块时,按照每个数据块的位置进行排序。
阅读全文
相关推荐
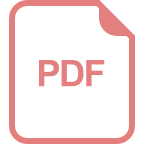


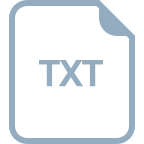
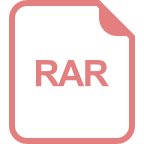
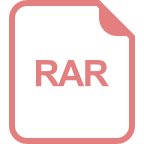
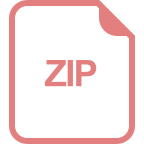
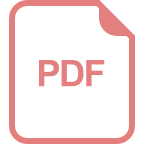
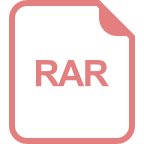
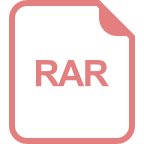
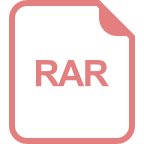
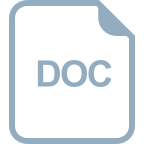
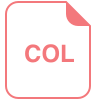
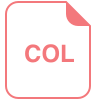
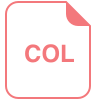
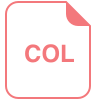
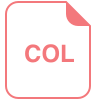