使用SpringBoot 实现购物车业务和订单业务 2.1 购物车基于redis实现, 建议用hash类型,方法包括但不限于: 添加商品到购物车/从购物车删除商品/修改购物车中的商品数量/统计总价 2.2 订单的异步生成: 当购物车提交时创建订单, 并用mq实现异步订单: 生成订单编号, 记录mq, 即可给前端返回
时间: 2023-03-13 09:46:39 浏览: 250
使用SpringBoot实现购物车业务和订单业务可以采取以下步骤:1)基于Redis实现购物车,采用Hash类型,实现添加商品到购物车、从购物车删除商品、修改购物车中商品数量以及统计总价;2)订单的异步生成:当购物车提交时创建订单,通过使用MQ来实现异步订单,生成订单编号、记录MQ,然后即可向前端返回成功信息。
相关问题
springboot redis 实现 购物车
实现购物车可以使用 Redis 来存储购物车的数据,Spring Boot 中可以使用 Spring Data Redis 来操作 Redis。
首先,需要添加 Redis 相关的依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
然后,需要在配置文件中配置 Redis 的连接信息:
```yaml
spring:
redis:
host: localhost
port: 6379
```
接下来,定义一个购物车项的类:
```java
public class CartItem {
private Long productId;
private Integer quantity;
// getters and setters
}
```
然后,定义一个购物车的服务类:
```java
@Service
public class CartService {
private final RedisTemplate<String, CartItem> redisTemplate;
public CartService(RedisTemplate<String, CartItem> redisTemplate) {
this.redisTemplate = redisTemplate;
}
public void addItem(String userId, Long productId, Integer quantity) {
String key = "cart:" + userId;
CartItem cartItem = redisTemplate.opsForHash().get(key, productId);
if (cartItem == null) {
cartItem = new CartItem();
cartItem.setProductId(productId);
cartItem.setQuantity(quantity);
} else {
cartItem.setQuantity(cartItem.getQuantity() + quantity);
}
redisTemplate.opsForHash().put(key, productId, cartItem);
}
public void removeItem(String userId, Long productId) {
String key = "cart:" + userId;
redisTemplate.opsForHash().delete(key, productId);
}
public List<CartItem> getItems(String userId) {
String key = "cart:" + userId;
Map<Object, Object> entries = redisTemplate.opsForHash().entries(key);
List<CartItem> items = new ArrayList<>(entries.size());
for (Map.Entry<Object, Object> entry : entries.entrySet()) {
CartItem cartItem = (CartItem) entry.getValue();
items.add(cartItem);
}
return items;
}
}
```
在这个购物车服务类中,我们使用 Redis 的 Hash 类型来存储购物车数据,其中键为用户 ID,值为一个 Hash,其中每个键值对表示一个购物车项。在 `addItem` 方法中,我们首先根据用户 ID 和商品 ID 获取原来的购物车项,如果购物车项不存在,则创建一个新的购物车项;否则,更新购物车项的数量。然后,使用 Redis 的 `opsForHash()` 方法将购物车项保存到 Redis 中。在 `removeItem` 方法中,我们使用 `opsForHash().delete()` 方法从 Redis 中删除购物车项。在 `getItems` 方法中,我们使用 `opsForHash().entries()` 方法获取购物车中所有的商品项,并将所有的购物车项转换成一个列表返回。
最后,我们可以在控制器中注入购物车服务类,然后调用其方法来实现购物车的增加、删除和查询:
```java
@RestController
public class CartController {
private final CartService cartService;
public CartController(CartService cartService) {
this.cartService = cartService;
}
@PostMapping("/cart")
public void addItem(@RequestParam String userId,
@RequestParam Long productId,
@RequestParam Integer quantity) {
cartService.addItem(userId, productId, quantity);
}
@DeleteMapping("/cart")
public void removeItem(@RequestParam String userId,
@RequestParam Long productId) {
cartService.removeItem(userId, productId);
}
@GetMapping("/cart")
public List<CartItem> getItems(@RequestParam String userId) {
return cartService.getItems(userId);
}
}
```
这样,我们就可以使用 Redis 实现购物车了。
使用redis的hash结构如何实现购物车的功能
使用Redis的Hash结构可以很方便地实现购物车的功能。下面是一个示例的购物车实现步骤:
1. 将每个商品作为Hash结构存储在Redis中,以商品ID作为Hash的key,商品信息(如名称、价格、数量等)作为Hash的field和value。
```
HSET cart:userid productid1 '{"name":"Product 1","price":10.99,"quantity":2}'
HSET cart:userid productid2 '{"name":"Product 2","price":15.99,"quantity":1}'
```
2. 当用户添加商品到购物车时,使用HSET命令将商品信息添加到购物车的Hash中。
```
HSET cart:userid productid3 '{"name":"Product 3","price":9.99,"quantity":3}'
```
3. 当用户需要更新购物车中的商品数量时,使用HSET命令更新对应商品的数量。
```
HSET cart:userid productid1 '{"name":"Product 1","price":10.99,"quantity":3}'
```
4. 当用户需要删除购物车中的商品时,使用HDEL命令删除对应商品的Hash。
```
HDEL cart:userid productid2
```
5. 当用户需要获取购物车中的所有商品时,使用HGETALL命令获取购物车的Hash,然后遍历获取每个商品的信息。
```
HGETALL cart:userid
```
这样,通过使用Redis的Hash结构,可以方便地存储和管理购物车中的商品信息。注意,这只是一个简单的示例,实际应用中可能还需要考虑用户身份、购物车过期时间等其他因素来完善购物车功能。
相关推荐
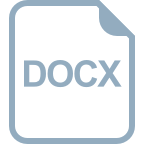
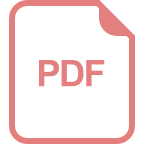
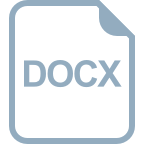
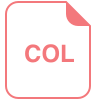
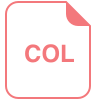
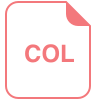
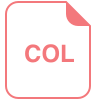
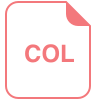




