用c语言设计算法对一个已知分块大小的分块有序表建立索引表,设计分块搜索算法。在一个已经建立索引表的分块有序表上搜索指定关键字值的记录
时间: 2024-05-04 20:19:06 浏览: 93
算法步骤如下:
1. 首先,将分块有序表按照分块大小划分为若干块,每一块内部有序,且每一块内的最大关键字值小于下一块的最小关键字值。
2. 对于每一块,取出其中最大的关键字值,将这些值存储在索引表中,形成一个单调不降的关键字序列。
3. 在索引表中使用二分查找算法,找到包含待查找记录的块的位置。
4. 在对应的块中使用顺序查找算法,找到关键字值等于待查找记录关键字值的记录,或者确定待查找记录不存在。
下面是C语言代码实现:
```c
#include <stdio.h>
#define BLOCK_SIZE 5 // 分块大小
// 分块有序表结构体
typedef struct {
int* data; // 数据存储区
int size; // 分块个数
} BlockList;
// 索引表结构体
typedef struct {
int* index; // 索引存储区
int size; // 索引个数
} IndexList;
// 初始化分块有序表
void init_block_list(BlockList* list, int arr[], int n) {
int i, j;
list->data = arr;
list->size = n / BLOCK_SIZE + (n % BLOCK_SIZE != 0); // 计算分块个数
for (i = 0; i < list->size; i++) {
// 对每一块进行插入排序
for (j = i * BLOCK_SIZE + 1; j < (i + 1) * BLOCK_SIZE && j < n; j++) {
int temp = arr[j];
int k = j - 1;
while (k >= i * BLOCK_SIZE && arr[k] > temp) {
arr[k + 1] = arr[k];
k--;
}
arr[k + 1] = temp;
}
}
}
// 初始化索引表
void init_index_list(BlockList* list, IndexList* index_list) {
int i;
index_list->index = (int*)malloc(sizeof(int) * list->size);
index_list->size = list->size;
for (i = 0; i < list->size; i++) {
// 取出每一块的最大值作为索引值
index_list->index[i] = list->data[(i + 1) * BLOCK_SIZE - 1];
}
}
// 在分块有序表上搜索指定关键字值的记录
int block_search(BlockList* list, IndexList* index_list, int key) {
int i, j;
// 在索引表中使用二分查找算法,找到包含待查找记录的块的位置
int left = 0, right = index_list->size - 1;
while (left <= right) {
int mid = (left + right) / 2;
if (index_list->index[mid] > key) {
right = mid - 1;
} else if (index_list->index[mid] < key) {
left = mid + 1;
} else {
return mid;
}
}
// 在对应的块中使用顺序查找算法
if (left == 0) {
i = 0;
} else {
i = left * BLOCK_SIZE;
}
j = (left + 1) * BLOCK_SIZE - 1;
while (i <= j && list->data[i] <= key) {
if (list->data[i] == key) {
return i;
}
i++;
}
return -1; // 未找到
}
int main() {
int arr[] = {4, 2, 1, 3, 5, 9, 7, 8, 6, 10};
int n = sizeof(arr) / sizeof(int);
BlockList list;
init_block_list(&list, arr, n);
IndexList index_list;
init_index_list(&list, &index_list);
int key = 7;
int pos = block_search(&list, &index_list, key);
if (pos == -1) {
printf("未找到 %d\n", key);
} else {
printf("找到 %d,下标为 %d\n", key, pos);
}
return 0;
}
```
阅读全文
相关推荐







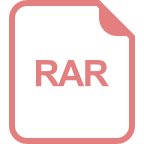
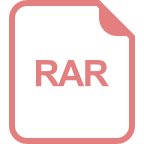

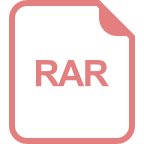



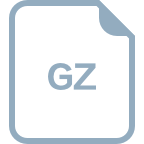



