使用netty实现多端口
时间: 2023-05-28 09:05:50 浏览: 668
使用Netty实现多端口可以通过创建多个ServerBootstrap实例,并分别绑定不同的端口。以下是一个示例代码:
```
public class MultiPortServer {
private final int port1;
private final int port2;
public MultiPortServer(int port1, int port2) {
this.port1 = port1;
this.port2 = port2;
}
public void run() throws Exception {
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b1 = new ServerBootstrap();
b1.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new MultiPortServerHandler("Port 1"));
}
});
ServerBootstrap b2 = new ServerBootstrap();
b2.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
public void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new MultiPortServerHandler("Port 2"));
}
});
b1.bind(port1).sync();
b2.bind(port2).sync();
} finally {
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
public static void main(String[] args) throws Exception {
int port1 = 8000;
int port2 = 8001;
new MultiPortServer(port1, port2).run();
}
}
public class MultiPortServerHandler extends SimpleChannelInboundHandler<ByteBuf> {
private final String serverName;
public MultiPortServerHandler(String serverName) {
this.serverName = serverName;
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
System.out.println(serverName + " active");
}
@Override
protected void channelRead0(ChannelHandlerContext ctx, ByteBuf in) {
System.out.println(serverName + " received: " + in.toString(CharsetUtil.UTF_8));
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close();
}
}
```
在上述示例中,通过创建两个ServerBootstrap实例,并分别绑定不同的端口。同时,每个ServerBootstrap实例都配置了自己的ChannelHandler。在MultiPortServerHandler中,可以根据serverName来区分不同的端口。当有数据到来时,会打印出数据来源的端口信息。
相关推荐
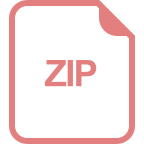














