Java中定义类函数,求圆柱体体积
时间: 2024-05-24 13:12:56 浏览: 18
public class Cylinder {
double radius;
double height;
public Cylinder(double r, double h) {
radius = r;
height = h;
}
public double getVolume() {
double volume = Math.PI * Math.pow(radius, 2) * height;
return volume;
}
}
// Example usage:
Cylinder c = new Cylinder(2.5, 5.0);
double volume = c.getVolume(); // volume = 98.17477...
相关问题
java定义一个圆柱体类cylinder
下面是一个简单的Java定义圆柱体类Cylinder的示例:
```
public class Cylinder {
private double radius;
private double height;
public Cylinder(double radius, double height) {
this.radius = radius;
this.height = height;
}
public double getRadius() {
return radius;
}
public void setRadius(double radius) {
this.radius = radius;
}
public double getHeight() {
return height;
}
public void setHeight(double height) {
this.height = height;
}
public double getBaseArea() {
return Math.PI * radius * radius;
}
public double getVolume() {
return getBaseArea() * height;
}
public double getSurfaceArea() {
return 2 * Math.PI * radius * height + 2 * getBaseArea();
}
public String toString() {
return "Cylinder [radius=" + radius + ", height=" + height + "]";
}
}
```
这个类包含了圆柱体的半径和高度属性,以及构造函数、访问器和更改器方法。
它还声明了三个方法来计算圆柱体的底面积、体积和表面积。toString方法用于将对象转换为字符串表示。
在使用这个类时,可以创建一个新的Cylinder对象,并对其属性进行设置:
```
Cylinder cyl = new Cylinder(5.0, 10.0);
cyl.setRadius(6.0);
cyl.setHeight(12.0);
```
然后,可以调用方法来计算圆柱体的底面积、体积和表面积:
```
double baseArea = cyl.getBaseArea();
double volume = cyl.getVolume();
double surfaceArea = cyl.getSurfaceArea();
```
输出Cylinder对象的字符串表示:
```
System.out.println(cyl.toString());
```
java类和对象之对象组合之求圆柱体积
### 回答1:
圆柱体体积公式为πr^2h,其中r为圆柱体的半径,h为圆柱体的高。
在java中,可以通过定义一个类来表示圆柱体,该类应该有半径和高两个属性,并且提供一个计算体积的方法。
例如:
```
public class Cylinder {
private double radius;
private double height;
public Cylinder(double radius, double height) {
this.radius = radius;
this.height = height;
}
public double getVolume() {
return Math.PI * Math.pow(radius, 2) * height;
}
}
```
在这个例子中,我们通过构造函数`Cylinder(double radius, double height)`创建圆柱体对象,并通过方法`getVolume()`计算体积。
### 回答2:
对于一个圆柱体,我们可以用两个基本的参数来描述它:底面半径和高度。底面半径是一个圆的半径,高度则是从底部到顶部的距离。为了在Java中描述一个圆柱体,我们需要使用类和对象的概念。
首先,我们需要定义一个“圆”对象,它包含圆的半径。可以使用下面的代码来定义一个“圆”对象:
class Circle {
// 定义圆的半径
double radius;
// 构造函数
public Circle(double radius) {
this.radius = radius;
}
// 计算圆的面积
public double getArea() {
return Math.PI * radius * radius;
}
}
接下来,我们可以定义一个“圆柱体”对象,它包含一个底面圆对象和圆柱体的高度。可以使用下面的代码来定义一个“圆柱体”对象:
class Cylinder {
// 定义底面圆对象
Circle base;
// 定义圆柱体的高度
double height;
// 构造函数
public Cylinder(Circle base, double height) {
this.base = base;
this.height = height;
}
// 计算圆柱体的体积
public double getVolume() {
return base.getArea() * height;
}
}
在上述代码中,我们使用了“对象组合”的概念,即在一个类中使用另一个类的对象。在“圆柱体”类中,我们使用“圆”对象来表示圆柱体的底面,这样就能够方便地计算圆柱体的体积了。
最后,我们可以使用下面的代码来创建一个圆柱体对象并计算其体积:
Circle c = new Circle(5.0);
Cylinder cy = new Cylinder(c, 10.0);
double volume = cy.getVolume();
System.out.println(volume);
在上述代码中,我们先创建一个“圆”对象c,并设置其半径为5.0。然后,我们创建一个“圆柱体”对象cy,其中底面圆对象为c,高度为10.0。最后,我们调用cy的getVolume()方法来计算圆柱体的体积,并输出结果。在这个例子中,圆柱体的体积为785.3981633974483,单位为立方单位。
### 回答3:
Java是一种面向对象的编程语言,其类和对象是Java编程中常用的基本概念。在Java中,一个类是一种数据类型,它定义了一组属性和方法,而一个对象则是该类的一个实例,具有类中定义的属性和方法。
在Java中,对象组合是指在一个类中使用另一个类的实例作为其属性。例如,我们可以定义一个圆柱体类,它包含一个圆类的实例和一个高度属性,通过这种组合方式来求解圆柱体的体积。
定义一个圆类:
public class Circle {
private double radius; // 圆半径
// 构造函数
public Circle(double r) {
radius = r;
}
// 获取圆面积
public double getArea() {
return Math.PI * radius * radius;
}
}
定义一个圆柱体类:
public class Cylinder {
private Circle base; // 圆底面
private double height; // 高度
// 构造函数
public Cylinder(double r, double h) {
base = new Circle(r); // 创建圆底面实例
height = h;
}
// 获取圆柱体体积
public double getVolume() {
return base.getArea() * height; // 底面积乘以高度
}
}
通过对象组合的方式,在圆柱体类中创建一个圆实例作为属性,即可使用圆的属性和方法来计算圆柱体的体积。在圆柱体类的构造函数中,通过创建圆实例并传递半径参数来初始化底面属性。在获取圆柱体体积时,调用圆的getArea()方法来获取底面积,再乘以高度属性即可。
通过上述代码示例,我们可以看出对象组合在Java编程中的实际应用。它可以避免重复编码,提高代码的复用性和可维护性,同时也更符合面向对象的编程思想。
相关推荐
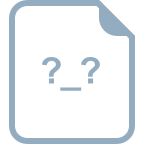










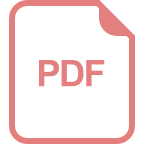
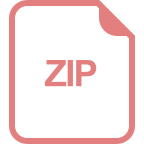