利用C++单链表写一个乐库管理系统
时间: 2023-12-24 19:03:42 浏览: 87
好的,下面是一个基于单链表的乐库管理系统的C++实现方案,供您参考:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义歌曲信息结构体
struct Song {
string name;
string artist;
string album;
int duration;
};
// 定义单链表节点结构体
struct SongNode {
Song song;
SongNode* next;
};
// 定义乐库管理系统类
class MusicLibrary {
public:
// 构造函数
MusicLibrary() {
head = NULL;
count = 0;
}
// 析构函数
~MusicLibrary() {
// 释放链表内存
SongNode* p = head;
while (p) {
SongNode* q = p->next;
delete p;
p = q;
}
}
// 添加歌曲
void addSong(Song song) {
SongNode* node = new SongNode;
node->song = song;
node->next = head;
head = node;
count++;
}
// 删除歌曲
void removeSong(string name) {
SongNode* p = head;
SongNode* q = NULL;
while (p) {
if (p->song.name == name) {
if (q) {
q->next = p->next;
} else {
head = p->next;
}
delete p;
count--;
return;
}
q = p;
p = p->next;
}
cout << "歌曲不存在" << endl;
}
// 修改歌曲信息
void updateSong(string name, Song song) {
SongNode* p = head;
while (p) {
if (p->song.name == name) {
p->song = song;
return;
}
p = p->next;
}
cout << "歌曲不存在" << endl;
}
// 按歌曲名称查找歌曲
Song* findSong(string name) {
SongNode* p = head;
while (p) {
if (p->song.name == name) {
return &(p->song);
}
p = p->next;
}
return NULL;
}
// 获取歌曲数量
int getCount() {
return count;
}
private:
SongNode* head;
int count;
};
// 测试代码
int main() {
MusicLibrary library;
// 添加几首歌曲
Song song1 = {"演员", "薛之谦", "初学者", 230};
Song song2 = {"绅士", "薛之谦", "绅士", 200};
Song song3 = {"认真的雪", "薛之谦", "认真的雪", 260};
library.addSong(song1);
library.addSong(song2);
library.addSong(song3);
cout << "当前歌曲数量:" << library.getCount() << endl;
// 查找歌曲
Song* pSong = library.findSong("绅士");
if (pSong) {
cout << "歌曲名称:" << pSong->name << endl;
cout << "歌手名称:" << pSong->artist << endl;
cout << "专辑名称:" << pSong->album << endl;
cout << "歌曲时长:" << pSong->duration << endl;
} else {
cout << "未找到歌曲" << endl;
}
// 修改歌曲信息
Song song4 = {"绅士", "薛之谦", "绅士(Live版)", 250};
library.updateSong("绅士", song4);
pSong = library.findSong("绅士");
if (pSong) {
cout << "歌曲名称:" << pSong->name << endl;
cout << "歌手名称:" << pSong->artist << endl;
cout << "专辑名称:" << pSong->album << endl;
cout << "歌曲时长:" << pSong->duration << endl;
} else {
cout << "未找到歌曲" << endl;
}
// 删除歌曲
library.removeSong("演员");
cout << "当前歌曲数量:" << library.getCount() << endl;
return 0;
}
```
该代码定义了一个MusicLibrary类,包含了添加歌曲、删除歌曲、修改歌曲信息、按歌曲名称查找歌曲等功能。该类内部使用单链表来存储歌曲信息。在测试代码中,我们添加了三首歌曲,然后查找歌曲、修改歌曲信息、删除歌曲,并输出当前歌曲数量。
阅读全文
相关推荐





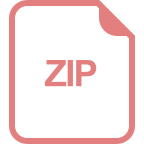
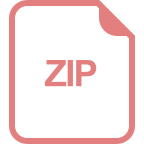
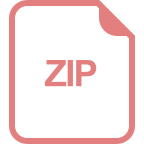





