import smtplib from email.mime.text import MIMEText from email.header import Header def send_pick_rubbish_message(body,subject): mail_host = "smtp.163.com" # 设置服务器 mail_user = "steam_robot_send@163.com" # 用户名 mail_pass = "UHGBXMHQUAYNYVHM" # 授权码,注意不是邮箱登录密码,是上述设置的授权密码!!! sender = 'steam_robot_send@163.com' #receivers = ['2499690965@qq.com','1272365872@qq.com','993592136@qq.com'] # 接收邮件,可设置为你的QQ邮箱或者其他邮箱 receivers = ['dyf2b2@163..com'] message = MIMEText(body, 'plain', 'utf-8') message['From'] = "steam_robot_send@163.com" message['To'] = "dyf2b2@163..com" message['Subject'] = Header(subject, 'utf-8') smtpObj = smtplib.SMTP() smtpObj.connect(mail_host, 25) smtpObj.set_debuglevel(1) smtpObj.login(mail_user, mail_pass) smtpObj.sendmail(sender, receivers, message.as_string()) print("邮件发送成功")
时间: 2024-03-14 18:48:43 浏览: 127
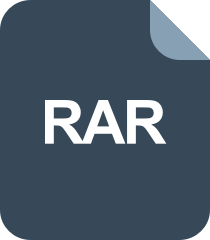
Examples_email.rar
这段代码是 Python 的邮件发送函数,其中 `smtplib` 库用于连接邮件服务器,`email.mime.text` 和 `email.header` 用于构建邮件消息。函数 `send_pick_rubbish_message` 的参数 `body` 和 `subject` 分别表示邮件正文和主题,其余的变量与值的含义与上面的代码相同。发送邮件的过程与上面的代码也相同,需要先设置好变量的值,然后调用函数即可。请注意,在发送邮件的过程中,需要设置正确的收件人地址和发件人地址,否则邮件将无法送达。
阅读全文
相关推荐
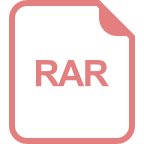
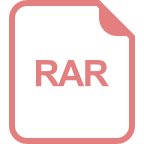







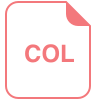
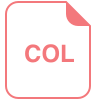
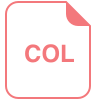
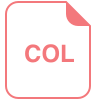


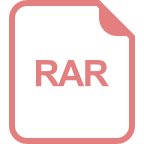
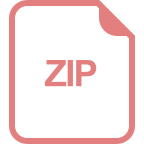