unity scrollview 无限循环代码
时间: 2023-08-06 15:24:48 浏览: 59
实现无限循环的 ScrollView,可以使用 Unity 的 UI 布局组件和代码结合的方式来实现。
首先,在 ScrollView 中添加一个 Content 布局组件,用于控制 ScrollView 中的子控件排列。
然后,在代码中动态生成需要显示的子控件,并将其添加到 Content 中。为了实现无限循环,需要在首尾各添加一个相同的子控件,这样在滑动到末尾时,可以无缝地切换到开头,实现循环。
以下是一个简单的示例代码,可以放在 ScrollView 的父物体上:
```csharp
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class InfiniteScrollView : MonoBehaviour
{
public GameObject itemPrefab;
public int itemCount = 10;
private List<GameObject> itemList = new List<GameObject>();
private RectTransform contentRectTransform;
private float itemHeight;
private int currentItemIndex = 0;
void Start()
{
// 获取 Content 的 RectTransform 组件
contentRectTransform = GetComponentInChildren<ScrollRect>().content;
// 获取子控件的高度
itemHeight = itemPrefab.GetComponent<RectTransform>().rect.height;
// 生成子控件
for (int i = 0; i < itemCount + 2; i++)
{
GameObject item = Instantiate(itemPrefab, contentRectTransform);
item.transform.localPosition = new Vector3(0, -itemHeight * i, 0);
itemList.Add(item);
}
// 更新 Content 的高度
contentRectTransform.sizeDelta = new Vector2(contentRectTransform.sizeDelta.x, itemHeight * (itemCount + 2));
// 更新子控件的内容
UpdateItemContent();
}
void Update()
{
// 判断是否需要切换子控件
if (Input.GetKeyDown(KeyCode.UpArrow))
{
currentItemIndex++;
if (currentItemIndex > itemCount + 1)
{
currentItemIndex = 1;
}
UpdateItemContent();
}
else if (Input.GetKeyDown(KeyCode.DownArrow))
{
currentItemIndex--;
if (currentItemIndex < 1)
{
currentItemIndex = itemCount + 1;
}
UpdateItemContent();
}
}
void UpdateItemContent()
{
// 更新子控件的内容
for (int i = 0; i < itemList.Count; i++)
{
int index = currentItemIndex - (itemList.Count - i - 1);
if (index <= 0)
{
index += itemCount;
}
else if (index > itemCount)
{
index -= itemCount;
}
itemList[i].GetComponentInChildren<Text>().text = "Item " + index;
}
// 更新 Content 的位置
contentRectTransform.anchoredPosition = new Vector2(contentRectTransform.anchoredPosition.x, itemHeight * (currentItemIndex - 1));
}
}
```
这个示例代码实现了一个简单的无限循环 ScrollView,可以根据实际需求进行修改。其中,itemPrefab 是用于生成子控件的预制体,itemCount 是需要显示的子控件个数。在 UpdateItemContent 函数中,根据当前的 currentItemIndex 来更新子控件的内容,并将 Content 移动到对应的位置。在 Update 函数中,通过监听上下箭头键来模拟手指滑动,实现切换子控件的效果。
相关推荐
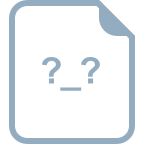
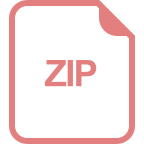
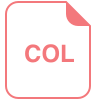
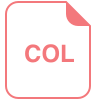
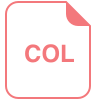
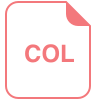
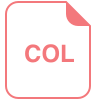









