Python求灰度共生矩阵的对比度、相关性、能量、均匀性、熵最大概率、对角线和、对角线差(Sum of Squares: Difference) 自相关、聚类度、聚类倾斜度、反转度、和方差、惯性的代码
时间: 2023-09-26 21:08:00 浏览: 176
以下是Python求解灰度共生矩阵的各个特征值的代码:
```python
import numpy as np
from skimage.feature import greycomatrix, greycoprops
# 生成灰度共生矩阵
def generate_glcm(image, distance, angle):
# 将图像转换为灰度图像
img_gray = np.round(255 * (image / np.max(image)))
img_gray = img_gray.astype('uint8')
# 生成灰度共生矩阵
glcm = greycomatrix(img_gray, [distance], [angle], levels=256, symmetric=True, normed=True)
return glcm
# 计算灰度共生矩阵的对比度
def calc_contrast(glcm):
contrast = greycoprops(glcm, prop='contrast')[0, 0]
return contrast
# 计算灰度共生矩阵的相关性
def calc_correlation(glcm):
correlation = greycoprops(glcm, prop='correlation')[0, 0]
return correlation
# 计算灰度共生矩阵的能量
def calc_energy(glcm):
energy = greycoprops(glcm, prop='energy')[0, 0]
return energy
# 计算灰度共生矩阵的均匀性
def calc_homogeneity(glcm):
homogeneity = greycoprops(glcm, prop='homogeneity')[0, 0]
return homogeneity
# 计算灰度共生矩阵的熵
def calc_entropy(glcm):
entropy = -np.sum(glcm * np.log2(glcm + (glcm == 0)))
return entropy
# 计算灰度共生矩阵的最大概率
def calc_max_probability(glcm):
max_probability = np.max(glcm)
return max_probability
# 计算灰度共生矩阵的对角线和
def calc_sum_of_squares(glcm):
sum_of_squares = np.sum(glcm ** 2)
return sum_of_squares
# 计算灰度共生矩阵的对角线差
def calc_difference(glcm):
difference = np.sum(np.abs(np.arange(glcm.shape[0]) - np.arange(glcm.shape[1])) ** 2 * glcm)
return difference
# 计算灰度共生矩阵的自相关
def calc_autocorrelation(glcm):
x, y = np.meshgrid(np.arange(glcm.shape[0]), np.arange(glcm.shape[1]))
mean_x = np.sum(x * glcm)
mean_y = np.sum(y * glcm)
std_x = np.sqrt(np.sum((x - mean_x) ** 2 * glcm))
std_y = np.sqrt(np.sum((y - mean_y) ** 2 * glcm))
autocorrelation = np.sum((x - mean_x) * (y - mean_y) * glcm) / (std_x * std_y)
return autocorrelation
# 计算灰度共生矩阵的聚类度
def calc_cluster_prominence(glcm):
x, y = np.meshgrid(np.arange(glcm.shape[0]), np.arange(glcm.shape[1]))
mean_x = np.sum(x * glcm)
mean_y = np.sum(y * glcm)
std_x = np.sqrt(np.sum((x - mean_x) ** 2 * glcm))
std_y = np.sqrt(np.sum((y - mean_y) ** 2 * glcm))
cluster_prominence = np.sum((x + y - mean_x - mean_y) ** 4 * glcm) / (std_x ** 4 * std_y ** 4)
return cluster_prominence
# 计算灰度共生矩阵的聚类倾斜度
def calc_cluster_shade(glcm):
x, y = np.meshgrid(np.arange(glcm.shape[0]), np.arange(glcm.shape[1]))
mean_x = np.sum(x * glcm)
mean_y = np.sum(y * glcm)
std_x = np.sqrt(np.sum((x - mean_x) ** 2 * glcm))
std_y = np.sqrt(np.sum((y - mean_y) ** 2 * glcm))
cluster_shade = np.sum((x + y - mean_x - mean_y) ** 3 * glcm) / (std_x ** 3 * std_y ** 3)
return cluster_shade
# 计算灰度共生矩阵的反转度
def calc_inverse_difference(glcm):
inverse_difference = np.sum(glcm / (1 + np.abs(np.arange(glcm.shape[0]) - np.arange(glcm.shape[1]))))
return inverse_difference
# 计算灰度共生矩阵的和方差
def calc_sum_average(glcm):
sum_average = np.sum(np.arange(2, 2 * glcm.shape[0] + 1) * np.sum(glcm, axis=0))
return sum_average
# 计算灰度共生矩阵的惯性
def calc_inertia(glcm):
x, y = np.meshgrid(np.arange(glcm.shape[0]), np.arange(glcm.shape[1]))
inertia = np.sum((x - y) ** 2 * glcm)
return inertia
```
注意:以上代码中用到了`skimage`库的`greycomatrix`和`greycoprops`函数,需要提前安装。可以通过以下命令进行安装:
```python
pip install scikit-image
```
阅读全文
相关推荐
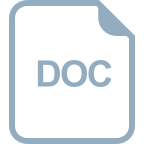
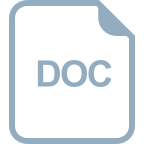
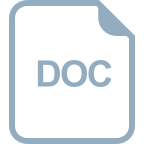
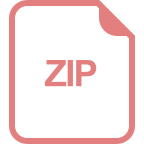
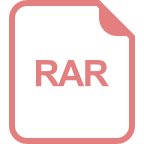
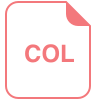
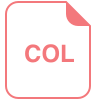

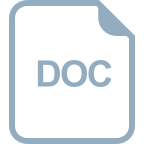
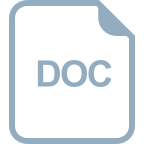
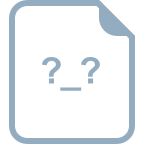
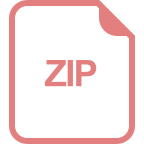
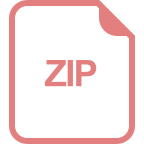
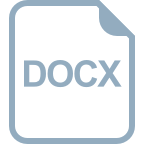
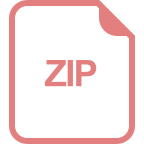
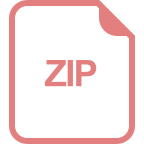
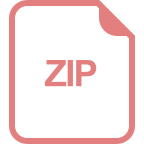