js generator函数
时间: 2023-11-23 08:57:14 浏览: 80
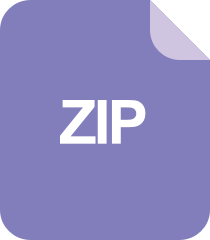
generator生成工具
Generator函数是ES6中的一种特殊函数,可以被暂停和恢复执行,可以生成一个迭代器对象。Generator函数使用function*关键字声明,内部使用yield语句来暂停函数执行并返回一个值,同时可以使用next()方法恢复函数执行并传入一个值作为上一个yield表达式的返回值。
下面是一个简单的Generator函数示例:
```javascript
function* myGenerator() {
yield 'apple';
yield 'banana';
yield 'orange';
}
const iterator = myGenerator();
console.log(iterator.next().value); // 输出:'apple'
console.log(iterator.next().value); // 输出:'banana'
console.log(iterator.next().value); // 输出:'orange'
console.log(iterator.next().value); // 输出:undefined
```
在上面的示例中,myGenerator函数使用yield语句来暂停函数执行并返回一个值。当调用next()方法时,函数会从上一个yield语句处恢复执行,并将传入的值作为上一个yield表达式的返回值。当函数执行到最后一个yield语句时,再调用next()方法会返回一个value属性为undefined的对象,表示生成器已经结束。
Generator函数可以用于异步编程,可以使用yield语句暂停异步操作的执行,等待异步操作完成后再恢复执行。下面是一个简单的异步操作的Generator函数示例:
```javascript
function* myAsyncGenerator() {
const response = yield fetch('https://jsonplaceholder.typicode.com/todos/1');
const json = yield response.json();
console.log(json);
}
const iterator = myAsyncGenerator();
const promise = iterator.next().value;
promise.then(response => iterator.next(response).value)
.then(json => iterator.next(json));
```
在上面的示例中,myAsyncGenerator函数首先使用yield语句暂停函数执行并返回一个promise对象,等待异步操作完成后再恢复执行。在调用next()方法时,我们可以通过Promise的then()方法获取异步操作的结果并传入next()方法,从而实现异步操作的暂停和恢复执行。
阅读全文
相关推荐
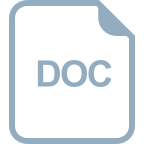
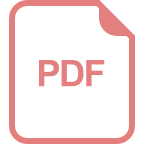
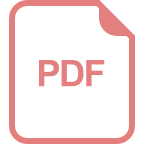
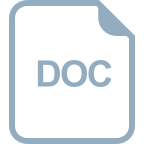
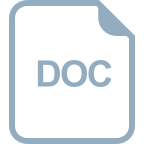
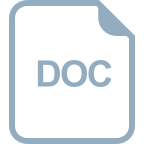
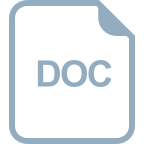
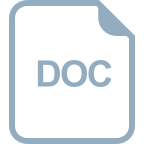
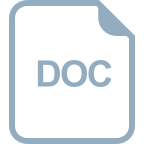
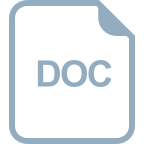
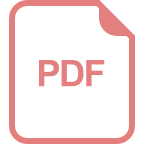
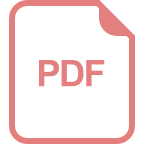
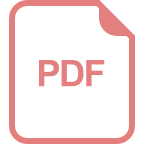
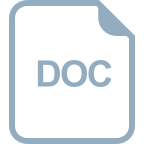
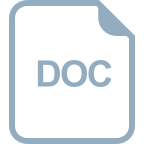
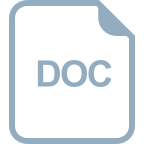
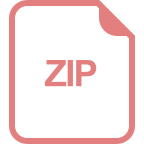